情景:
1.在使用MyBatis执行SQL(包含分页功能)的时候,明明SQL里没写LIMIT,执行时却多出了一个LiMIT。
2.在使用MyBatis执行SQL的时候,明明SQL里写的是SELECT * ...,执行时却执行了SELECT count(0) ...,后文中对此Bug进行说明。
解决方案:分页查询数据之前先清理分页缓存。
先执行
PageHelper.clearPage();
SQL代码如下:发现SQL中并没有LIMIT。
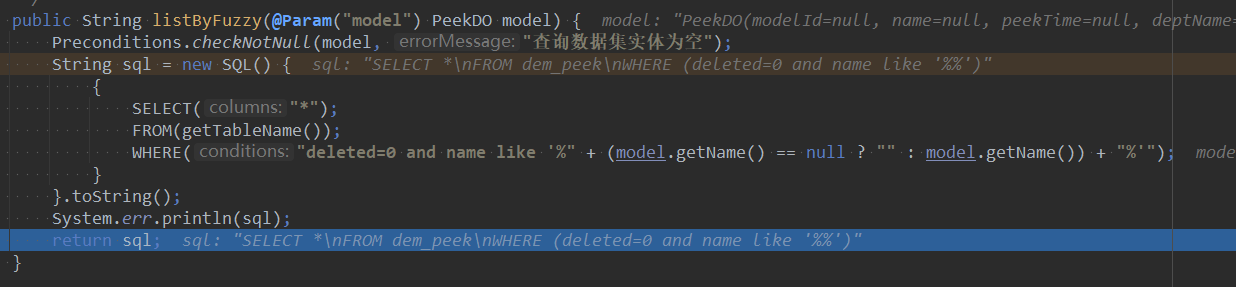
查询结果仅一页,期望的结果是多页。
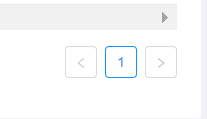
查看执行的SQL日志,从控制台中发现SQL中也是没有LIMIT 10,但是为什么在执行中多出了一个LIMIT ?呢?且这个LIMIT的参数就是Parameters的值(我设置的是10)。

参考了一下MyBatis官网和其他博客发现需要清理一下之前设置过的Page缓存,调用PgageHelper.clearPage()清理一下page缓存即可。
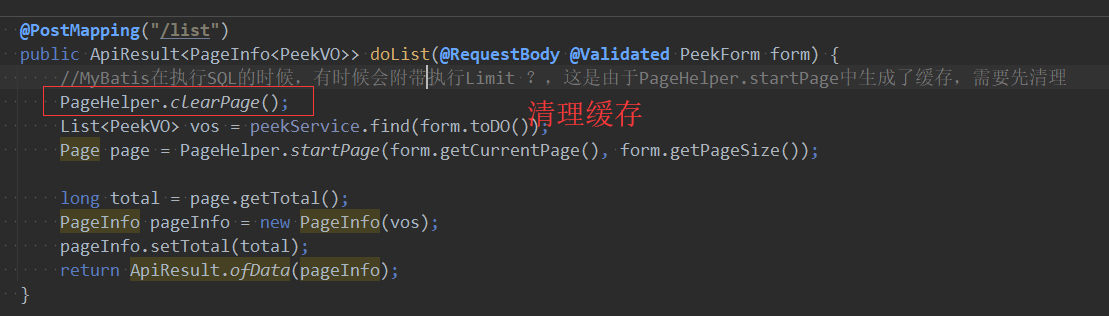
加入以上代码以后问题完美解决。LIMIT也消失了。

查看前端页面分页显示也正常了。
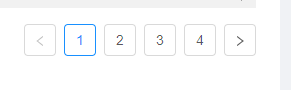
参考GitHub官网:MyBatis分页插件
https://github.com/pagehelper/Mybatis-PageHelper
MyBatis自动执行SELECT count(0) ...的Bug的解决方案:
正常情况下,执行的SQL如下:

出Bug时执行的SQL如下:

解决方案:添加PageHelper.clearPage();
附 PageHelper.clearPage()的代码。
package com.github.pagehelper.page;
import com.github.pagehelper.ISelect;
import com.github.pagehelper.Page;
import com.github.pagehelper.util.PageObjectUtil;
import java.util.Properties;
/**
* 基础分页方法
*
* @author liuzh
*/
public abstract class PageMethod {
protected static final ThreadLocal<Page> LOCAL_PAGE = new ThreadLocal<Page>();
protected static boolean DEFAULT_COUNT = true;
/**
* 设置 Page 参数
*
* @param page
*/
protected static void setLocalPage(Page page) {
LOCAL_PAGE.set(page);
}
/**
* 获取 Page 参数
*
* @return
*/
public static <T> Page<T> getLocalPage() {
return LOCAL_PAGE.get();
}
/**
* 移除本地变量
*/
public static void clearPage() {
LOCAL_PAGE.remove();
}
/**
* 获取任意查询方法的count总数
*
* @param select
* @return
*/
public static long count(ISelect select) {
Page<?> page = startPage(1, -1, true);
select.doSelect();
return page.getTotal();
}
/**
* 开始分页
*
* @param params
*/
public static <E> Page<E> startPage(Object params) {
Page<E> page = PageObjectUtil.getPageFromObject(params, true);
//当已经执行过orderBy的时候
Page<E> oldPage = getLocalPage();
if (oldPage != null && oldPage.isOrderByOnly()) {
page.setOrderBy(oldPage.getOrderBy());
}
setLocalPage(page);
return page;
}
/**
* 开始分页
*
* @param pageNum 页码
* @param pageSize 每页显示数量
*/
public static <E> Page<E> startPage(int pageNum, int pageSize) {
return startPage(pageNum, pageSize, DEFAULT_COUNT);
}
/**
* 开始分页
*
* @param pageNum 页码
* @param pageSize 每页显示数量
* @param count 是否进行count查询
*/
public static <E> Page<E> startPage(int pageNum, int pageSize, boolean count) {
return startPage(pageNum, pageSize, count, null, null);
}
/**
* 开始分页
*
* @param pageNum 页码
* @param pageSize 每页显示数量
* @param orderBy 排序
*/
public static <E> Page<E> startPage(int pageNum, int pageSize, String orderBy) {
Page<E> page = startPage(pageNum, pageSize);
page.setOrderBy(orderBy);
return page;
}
/**
* 开始分页
*
* @param pageNum 页码
* @param pageSize 每页显示数量
* @param count 是否进行count查询
* @param reasonable 分页合理化,null时用默认配置
* @param pageSizeZero true且pageSize=0时返回全部结果,false时分页,null时用默认配置
*/
public static <E> Page<E> startPage(int pageNum, int pageSize, boolean count, Boolean reasonable, Boolean pageSizeZero) {
Page<E> page = new Page<E>(pageNum, pageSize, count);
page.setReasonable(reasonable);
page.setPageSizeZero(pageSizeZero);
//当已经执行过orderBy的时候
Page<E> oldPage = getLocalPage();
if (oldPage != null && oldPage.isOrderByOnly()) {
page.setOrderBy(oldPage.getOrderBy());
}
setLocalPage(page);
return page;
}
/**
* 开始分页
*
* @param offset 起始位置,偏移位置
* @param limit 每页显示数量
*/
public static <E> Page<E> offsetPage(int offset, int limit) {
return offsetPage(offset, limit, DEFAULT_COUNT);
}
/**
* 开始分页
*
* @param offset 起始位置,偏移位置
* @param limit 每页显示数量
* @param count 是否进行count查询
*/
public static <E> Page<E> offsetPage(int offset, int limit, boolean count) {
Page<E> page = new Page<E>(new int[]{offset, limit}, count);
//当已经执行过orderBy的时候
Page<E> oldPage = getLocalPage();
if (oldPage != null && oldPage.isOrderByOnly()) {
page.setOrderBy(oldPage.getOrderBy());
}
setLocalPage(page);
return page;
}
/**
* 排序
*
* @param orderBy
*/
public static void orderBy(String orderBy) {
Page<?> page = getLocalPage();
if (page != null) {
page.setOrderBy(orderBy);
} else {
page = new Page();
page.setOrderBy(orderBy);
page.setOrderByOnly(true);
setLocalPage(page);
}
}
/**
* 设置参数
*
* @param properties 插件属性
*/
protected static void setStaticProperties(Properties properties){
//defaultCount,这是一个全局生效的参数,多数据源时也是统一的行为
if(properties != null){
DEFAULT_COUNT = Boolean.valueOf(properties.getProperty("defaultCount", "true"));
}
}
}
LOCAL_PAGE.remove()代码:
/**
* Removes the current thread's value for this thread-local
* variable. If this thread-local variable is subsequently
* {@linkplain #get read} by the current thread, its value will be
* reinitialized by invoking its {@link #initialValue} method,
* unless its value is {@linkplain #set set} by the current thread
* in the interim. This may result in multiple invocations of the
* {@code initialValue} method in the current thread.
*
* @since 1.5
*/
public void remove() {
ThreadLocalMap m = getMap(Thread.currentThread());
if (m != null)
m.remove(this);
}
官方的意思是删除此线程本地的当前线程的值变量。如果这个线程局部变量是随后的当前线程的{@linkplain #get read},其值为通过调用其{@link #initialValue}方法重新初始化,除非当前线程的值是{@linkplain #set set}在此期间。方法的多次调用当前线程中的{@code initialValue}。
来看看ThreadLocalMap的remove方法:
/**
* This class provides thread-local variables. These variables differ from
* their normal counterparts in that each thread that accesses one (via its
* {@code get} or {@code set} method) has its own, independently initialized
* copy of the variable. {@code ThreadLocal} instances are typically private
* static fields in classes that wish to associate state with a thread (e.g.,
* a user ID or Transaction ID).
*
* <p>For example, the class below generates unique identifiers local to each
* thread.
* A thread's id is assigned the first time it invokes {@code ThreadId.get()}
* and remains unchanged on subsequent calls.
* <pre>
* import java.util.concurrent.atomic.AtomicInteger;
*
* public class ThreadId {
* // Atomic integer containing the next thread ID to be assigned
* private static final AtomicInteger nextId = new AtomicInteger(0);
*
* // Thread local variable containing each thread's ID
* private static final ThreadLocal<Integer> threadId =
* new ThreadLocal<Integer>() {
* @Override protected Integer initialValue() {
* return nextId.getAndIncrement();
* }
* };
*
* // Returns the current thread's unique ID, assigning it if necessary
* public static int get() {
* return threadId.get();
* }
* }
* </pre>
* <p>Each thread holds an implicit reference to its copy of a thread-local
* variable as long as the thread is alive and the {@code ThreadLocal}
* instance is accessible; after a thread goes away, all of its copies of
* thread-local instances are subject to garbage collection (unless other
* references to these copies exist).
*
* @author Josh Bloch and Doug Lea
* @since 1.2
*/
public class ThreadLocal<T> {
/**
* Removes the current thread's value for this thread-local
* variable. If this thread-local variable is subsequently
* {@linkplain #get read} by the current thread, its value will be
* reinitialized by invoking its {@link #initialValue} method,
* unless its value is {@linkplain #set set} by the current thread
* in the interim. This may result in multiple invocations of the
* {@code initialValue} method in the current thread.
*
* @since 1.5
*/
public void remove() {
ThreadLocalMap m = getMap(Thread.currentThread());
if (m != null)
m.remove(this);
}
/**
* Get the map associated with a ThreadLocal. Overridden in
* InheritableThreadLocal.
*
* @param t the current thread
* @return the map
*/
ThreadLocalMap getMap(Thread t) {
return t.threadLocals;
}
static class ThreadLocalMap {
/**
* Remove the entry for key.
*/
private void remove(ThreadLocal<?> key) {
Entry[] tab = table;
int len = tab.length;
int i = key.threadLocalHashCode & (len-1);
for (Entry e = tab[i];
e != null;
e = tab[i = nextIndex(i, len)]) {
if (e.get() == key) {
e.clear();
expungeStaleEntry(i);
return;
}
}
}
}
}
官网的解释是:ThreadLocal 这个类提供线程局部变量。这些变量与它们的普通对应变量的不同之处在于,每个访问一个变量的线程(通过其{@code get}或{@code set}方法)都有自己的、独立初始化的变量副本。实例通常是类中希望将状态与线程(例如,用户ID或事务ID)关联的私有静态字段。
remove方法 删除此线程局部变量的当前线程值。如果这个线程局部变量随后被当前线程{@linkplain #get read}所读取,它的值将通过调用它的{@link #initialValue}方法重新初始化,除非它的值在过渡期间被当前线程为{@linkplain #set set}。这可能导致在当前线程中多次调用{@code initialValue}方法。
ThreadLocal是一个线程内部的数据存储类,通过它可以在当前线程存储数据,其内部维护了一个ThreadLocalMap,其内部Entity继承WeakReference,key是当前threadLocal对象。
使用场景:
当某些数据是以线程为作用域并且不同线程具有不同的数据副本的时候
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)