文章目录
- 前言
- Linux的API
- 获取时间
- *time函数*
- *gettimeofday函数*
- time函数、gettimeofday函数使用代码示例
- 时间转换
- *ctime函数*
- ctime函数使用代码示例
- *asctime函数*
- asctime函数使用代码示例
- 时间的分解与合成
-
- 总结
前言
在linux系统里面,我们有时候需要获取当前时间,用它来统计程序运行的时间,或者显示标准格式的日期和时间等等。那么怎么获取时间呢?下面就来了解一下。
Linux的API
获取时间
time函数
首先看看它在man 第2册中的介绍:
NAME
time - get time in seconds
SYNOPSIS
#include <time.h>
time_t time(time_t *tloc);
DESCRIPTION
time() returns the time as the number of seconds since the Epoch, 1970-01-01 00:00:00 +0000 (UTC).
If tloc is non-NULL, the return value is also stored in the memory pointed to by tloc.
RETURN VALUE
On success, the value of time in seconds since the Epoch is returned. On error, ((time_t) -1) is returned, and errno is set appro‐priately.
概括一下,如下表:
函数原型 | time_t time(time_t *tloc); | 备注 |
---|
要包含的头文件 | #include <time.h> | |
形参 | time_t *tloc | 指针非空就把时间值放入指针指向的内存;指针为空就忽略 |
返回值 | 成功:当前时间(单位为秒)/ 失败:((time_t) -1) | 返回的时间是从1970-01-01 00:00:00 +0000 (UTC)开始算起间隔的秒数 |
再啰嗦一遍: time函数,返回自Epoch以来的秒数,这是肯定的,同时可以接受一个time_t*类型的指针作为形参,执行该函数将会把这个秒数放入到指针所指向的位置 ,如果为NULL当然不用管了;
gettimeofday函数
函数原型:
include <sys/time.h>
int gettimeofday(struct timeval *tv, struct timezone *tz);
第一个参数 是一个结构体:
struct timeval {
time_t tv_sec; /* seconds /
suseconds_t tv_usec; / microseconds */
};
里面有秒数和微秒数,都是自Epoch以来的秒数。
第二个参数是一个时区结构,已经过时了,所以tz参数通常应该指定为NULL。
该函数会把自Epoch以来的秒数和微秒数放到tv结构体。
函数调用成功返回0,失败返回-1,并设置errno。
time函数、gettimeofday函数使用代码示例
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/time.h>
#include <time.h>
int main()
{
time_t tt;
struct timeval tv;
printf("Time Ret: %ld\n",time(NULL));
printf("Time Ret: %ld\n",time(&tt));
printf("Time Arg: %ld\n",tt);
int ret=gettimeofday(&tv,NULL);
printf("tv.tv_sec: %ld\n",tv.tv_sec);
printf("tv.tv_usec: %ld\n",tv.tv_usec);
return 0;
}
时间转换
问: 为什么要转换时间?
答:因为得到的时间是自Epoch以来的秒数,根本看不懂,于是需要把它转换为标准格式的日期和时间。
下面的函数可以实现这个功能。
ctime函数
最简单的是ctime函数,直接给返回一个长达26字节的字符串,包含了标准格式的日期和时间。
ctime函数使用代码示例
int main()
{
time_t tt;
tt=time(NULL);
printf("%s\n",ctime(&tt));
return 0;
}
运行效果如下,下方是作为对比的date命令。
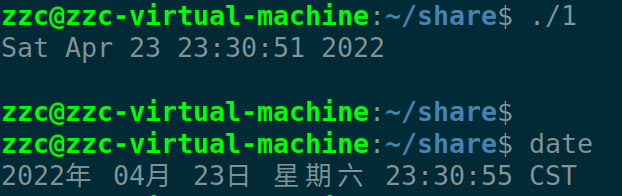
asctime函数
原型如下:
char *asctime(const struct tm *tm);
这个函数可以把任意一个tm结构体转换为标准格式的日期和时间。
asctime函数使用代码示例
#include <stdio.h>
#include <time.h>
int main()
{
time_t tt;
struct tm* ptm;
char *p;
tt=time(NULL);
ptm=gmtime(&tt);
p = asctime(ptm);
printf("now time is :%s\n", p);
return 0;
}
运行效果如下:

时间的分解与合成
时间的分解
有时候我们并不需要这么多的时间信息,例如我只想检查一下现在时间是否介于晚上7点到早上6点之间,然后决定是否打开路灯这样的工作,显然这个就需要提取时间中的各个不同要素。
可以使用下面这个函数把时间分解,
struct tm *gmtime(const time_t *timep);
首先是结构体 tm
struct tm {
int tm_sec;
int tm_min;
int tm_hour;
int tm_mday;
int tm_mon;
int tm_year;
int tm_wday;
int tm_yday;
int tm_isdst;
};
可以看到这个结构体中包含了最基本的年月日、时分秒、星期,还有DST(夏令时)等。
更有意思的是,秒并不是从0到59,而是从0~60,这是为了考虑闰秒的问题。另外,年并不是直接是年,而是从1900开始的偏移量,所以如果今年是2022年的话,这个字段得到的结果是122。
什么是夏令时?
夏令时,表示为了节约能源,人为规定时间的意思。也叫夏时制,夏令时(Daylight Saving
Time:DST),又称“日光节约时制”和“夏令时间”,在这一制度实行期间所采用的统一时间称为“夏令时间”。一般在天亮早的夏季人为将时间调快一小时,可以使人早起早睡,减少照明量,以充分利用光照资源,从而节约照明用电。各个采纳夏时制的国家具体规定不同。全世界有近110个国家每年要实行夏令时。
1986年4月,中国中央有关部门发出“在全国范围内实行夏时制的通知”,具体做法是:每年从四月中旬第一个星期日的凌晨2时整(北京时间),将时钟拨快一小时,即将表针由2时拨至3时,夏令时开始;到九月中旬第一个星期日的凌晨2时整(北京夏令时),再将时钟拨回一小时,即将表针由2时拨至1时,夏令时结束。从1986年到1991年的六个年度,除1986年因是实行夏时制的第一年,从5月4日开始到9月14日结束外,其它年份均按规定的时段施行。在夏令时开始和结束前几天,新闻媒体均刊登有关部门的通告。1992年起,夏令时暂停实行。
测试代码如下:
int main()
{
time_t tt;
struct tm* ptm;
tt=time(NULL);
printf("%s",ctime(&tt));
ptm=gmtime(&tt);
printf("Hour: %d\n",ptm->tm_hour);
return 0;
}
测试结果如下:
zzc@zzc-virtual-machine:~/share$ ./1
Sun Apr 24 00:00:30 2022
Hour: 16, DST:0
从结果来看,为什么ctime返回的时间是凌晨0点(24时),而gmtime返回的时间是16时?
因为ctime返回的是本地时间(这里是北京时间),而gmtime返回的是GMT时间(格林威治标准时间);北京时间是GMT+8,比格林威治标准时间早了8小时。
如果想直接分解成本地时间,那就把gmtime换成localtime。
localtime的函数原型:
struct tm *localtime(const time_t *timep);
时间的合成
时间的合成是时间的分解的逆向操作,所使用的函数是mktime,可以猜到,这个函数的参数是一个tm的结构体(或指针),返回值是time_t类型,函数原型如下:
time_t mktime(struct tm *tm);
总结
本文详细介绍了linux系统下有关时间的函数。
获取时间可以使用time、gettimeofday;
转换时间可以使用asctime、ctime;
分解时间可以使用gmtime、localtime;
合成时间可以使用mktime
感谢您的阅读,如有错误,欢迎批评指正。
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)