使用新建好的工程,见上一篇博文:使用CCS给TM4C123系列MCU新建工程
第一步:在自己的工程下新建一个FreeRTOS文件夹,在这个文件夹下新建两个文件夹:src和port
第二步:将include文件复制到工程文件夹中

第三步:将以下的文件复制到工程的src文件夹中
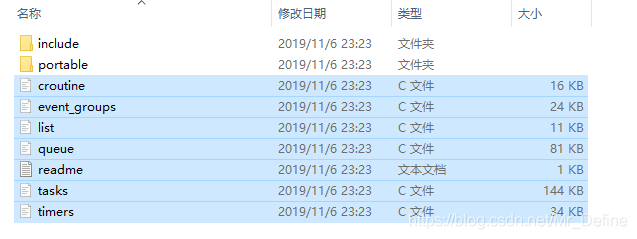
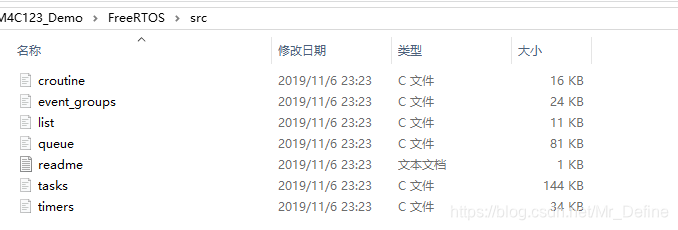
第四步:复制C:\ti\TivaWare_C_Series-2.1.4.178\third_party\FreeRTOS\Source\portable下的CCS和MemMang文件夹下的heap4.c两个文件到我们建好的的port文件夹中,这里用CCS代替RVDS。
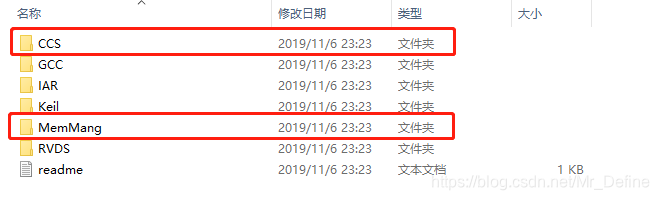
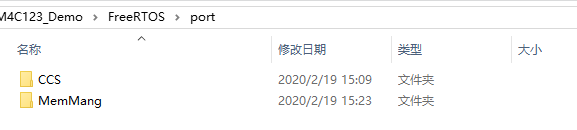
第五步:将C:\ti\TivaWare_C_Series-2.1.4.178\examples\boards\ek-tm4c123gxl\freertos_demo文件夹下的FreeRTOSConfig.h复制到工程中
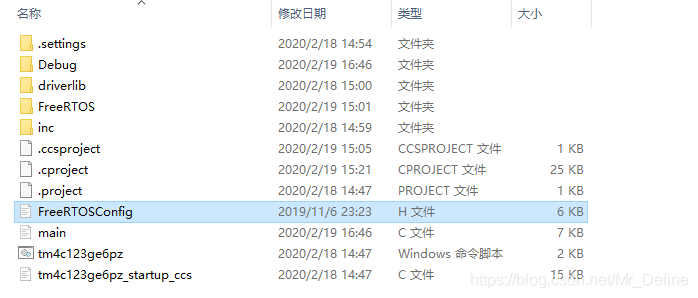
第六步:在CCS中增加头文件的路径
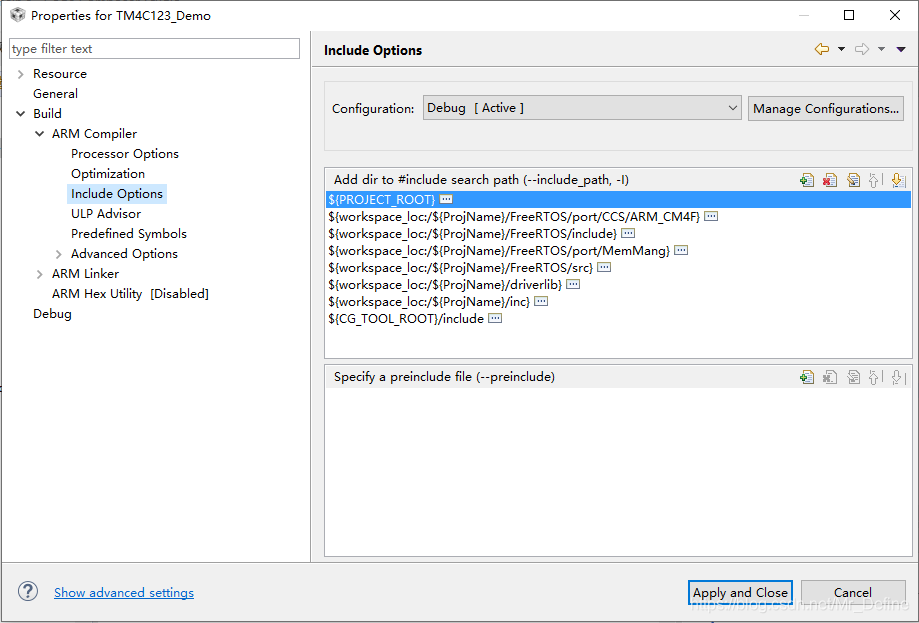
因为使用了硬件浮点单元,还要增加一个宏定义
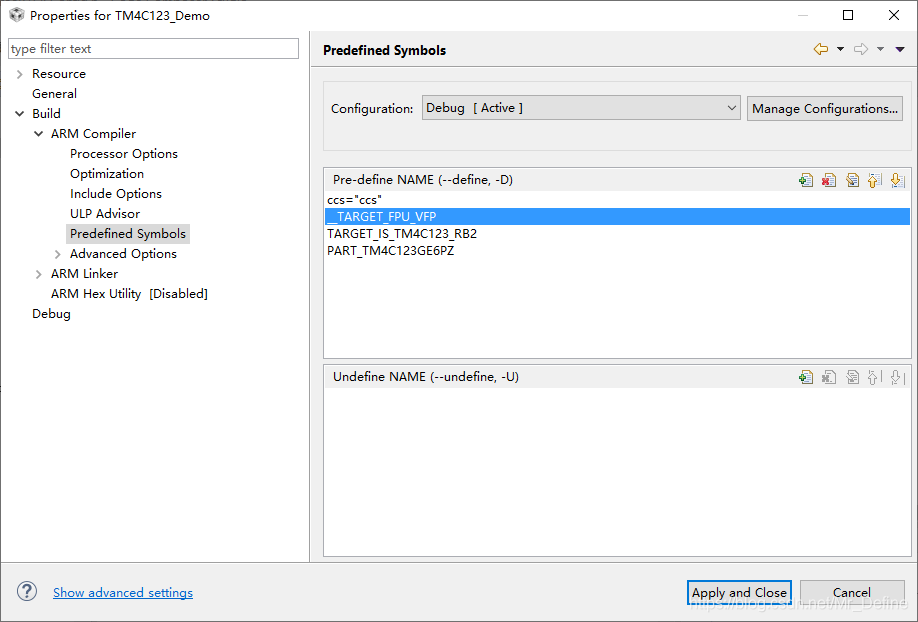
第七步:复制例程中的程序,屏蔽掉一我们没有的文件,测试编译是否有问题。
//*****************************************************************************
//
// freertos_demo.c - Simple FreeRTOS example.
//
// Copyright (c) 2012-2017 Texas Instruments Incorporated. All rights reserved.
// Software License Agreement
//
// Texas Instruments (TI) is supplying this software for use solely and
// exclusively on TI's microcontroller products. The software is owned by
// TI and/or its suppliers, and is protected under applicable copyright
// laws. You may not combine this software with "viral" open-source
// software in order to form a larger program.
//
// THIS SOFTWARE IS PROVIDED "AS IS" AND WITH ALL FAULTS.
// NO WARRANTIES, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT
// NOT LIMITED TO, IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
// A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. TI SHALL NOT, UNDER ANY
// CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR CONSEQUENTIAL
// DAMAGES, FOR ANY REASON WHATSOEVER.
//
// This is part of revision 2.1.4.178 of the EK-TM4C123GXL Firmware Package.
//
//*****************************************************************************
#include <stdbool.h>
#include <stdint.h>
#include "inc/hw_memmap.h"
#include "inc/hw_types.h"
#include "driverlib/gpio.h"
#include "driverlib/pin_map.h"
#include "driverlib/rom.h"
#include "driverlib/sysctl.h"
#include "driverlib/uart.h"
#include "FreeRTOS.h"
#include "task.h"
#include "queue.h"
#include "semphr.h"
//*****************************************************************************
//
//! \addtogroup example_list
//! <h1>FreeRTOS Example (freertos_demo)</h1>
//!
//! This application demonstrates the use of FreeRTOS on Launchpad.
//!
//! The application blinks the user-selected LED at a user-selected frequency.
//! To select the LED press the left button and to select the frequency press
//! the right button. The UART outputs the application status at 115,200 baud,
//! 8-n-1 mode.
//!
//! This application utilizes FreeRTOS to perform the tasks in a concurrent
//! fashion. The following tasks are created:
//!
//! - An LED task, which blinks the user-selected on-board LED at a
//! user-selected rate (changed via the buttons).
//!
//! - A Switch task, which monitors the buttons pressed and passes the
//! information to LED task.
//!
//! In addition to the tasks, this application also uses the following FreeRTOS
//! resources:
//!
//! - A Queue to enable information transfer between tasks.
//!
//! - A Semaphore to guard the resource, UART, from access by multiple tasks at
//! the same time.
//!
//! - A non-blocking FreeRTOS Delay to put the tasks in blocked state when they
//! have nothing to do.
//!
//! For additional details on FreeRTOS, refer to the FreeRTOS web page at:
//! http://www.freertos.org/
//
//*****************************************************************************
//*****************************************************************************
//
// The mutex that protects concurrent access of UART from multiple tasks.
//
//*****************************************************************************
xSemaphoreHandle g_pUARTSemaphore;
//*****************************************************************************
//
// The error routine that is called if the driver library encounters an error.
//
//*****************************************************************************
#ifdef DEBUG
void
__error__(char *pcFilename, uint32_t ui32Line)
{
}
#endif
//*****************************************************************************
//
// This hook is called by FreeRTOS when an stack overflow error is detected.
//
//*****************************************************************************
void
vApplicationStackOverflowHook(xTaskHandle *pxTask, char *pcTaskName)
{
//
// This function can not return, so loop forever. Interrupts are disabled
// on entry to this function, so no processor interrupts will interrupt
// this loop.
//
while(1)
{
}
}
//*****************************************************************************
//
// Configure the UART and its pins. This must be called before UARTprintf().
//
//*****************************************************************************
void
ConfigureUART(void)
{
//
// Enable the GPIO Peripheral used by the UART.
//
ROM_SysCtlPeripheralEnable(SYSCTL_PERIPH_GPIOA);
//
// Enable UART0
//
ROM_SysCtlPeripheralEnable(SYSCTL_PERIPH_UART0);
//
// Configure GPIO Pins for UART mode.
//
ROM_GPIOPinConfigure(GPIO_PA0_U0RX);
ROM_GPIOPinConfigure(GPIO_PA1_U0TX);
ROM_GPIOPinTypeUART(GPIO_PORTA_BASE, GPIO_PIN_0 | GPIO_PIN_1);
//
// Use the internal 16MHz oscillator as the UART clock source.
//
// UARTClockSourceSet(UART0_BASE, UART_CLOCK_PIOSC);
//
// //
// // Initialize the UART for console I/O.
// //
// UARTStdioConfig(0, 115200, 16000000);
}
//*****************************************************************************
//
// Initialize FreeRTOS and start the initial set of tasks.
//
//*****************************************************************************
int
main(void)
{
//
// Set the clocking to run at 50 MHz from the PLL.
//
ROM_SysCtlClockSet(SYSCTL_SYSDIV_4 | SYSCTL_USE_PLL | SYSCTL_XTAL_16MHZ |
SYSCTL_OSC_MAIN);
//
// Initialize the UART and configure it for 115,200, 8-N-1 operation.
//
ConfigureUART();
//
// Print demo introduction.
//
// UARTprintf("\n\nWelcome to the EK-TM4C123GXL FreeRTOS Demo!\n");
//
// Create a mutex to guard the UART.
//
g_pUARTSemaphore = xSemaphoreCreateMutex();
//
// Create the LED task.
//
// if(LEDTaskInit() != 0)
// {
//
// while(1)
// {
// }
// }
//
// Create the switch task.
//
// if(SwitchTaskInit() != 0)
// {
//
// while(1)
// {
// }
// }
//
// Start the scheduler. This should not return.
//
vTaskStartScheduler();
//
// In case the scheduler returns for some reason, print an error and loop
// forever.
//
while(1)
{
}
}
编译成功。
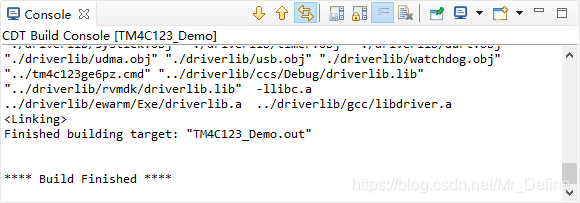
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)