目的:因为需要保存一个大大的.mp4视频,以防过程中设备出现异常导致整个长长的视频无法正常保存,所以采用分段保存视频的方式,每500帧保存一段,然后再将视频合到一起.最近刚开始学习python,发现python真的很好用,所以这次就使用python中的moviepy库来完成视频的合并.
一.安装moviepy
1. 你首先尝试使用 pip install moviepy指令是否可以正常安装moviepy库(我在python2.7上和python3.7上都尝试了这中安装方式都安装不了,所以不得不采用下面这个方式)
2.采用source文件安装.参照 https://blog.csdn.net/ucsheep/article/details/81000982 下载这个库的source文件,然后按照目录下的 README.rst 的指示安装,
首先cd到你下载的目录文件下,顺序执行
$ (sudo) pip install ez_setup
$ (sudo) python setup.py install
如果有错误提示
ERROR: moviepy 1.0.3 has requirement imageio<2.5,>=2.0, but you'll have imageio 2.8.0 which is incompatible.
那么就执行
pip install imageio
二.使用moviepy库合并多个视频
我的目录框架是这样的,
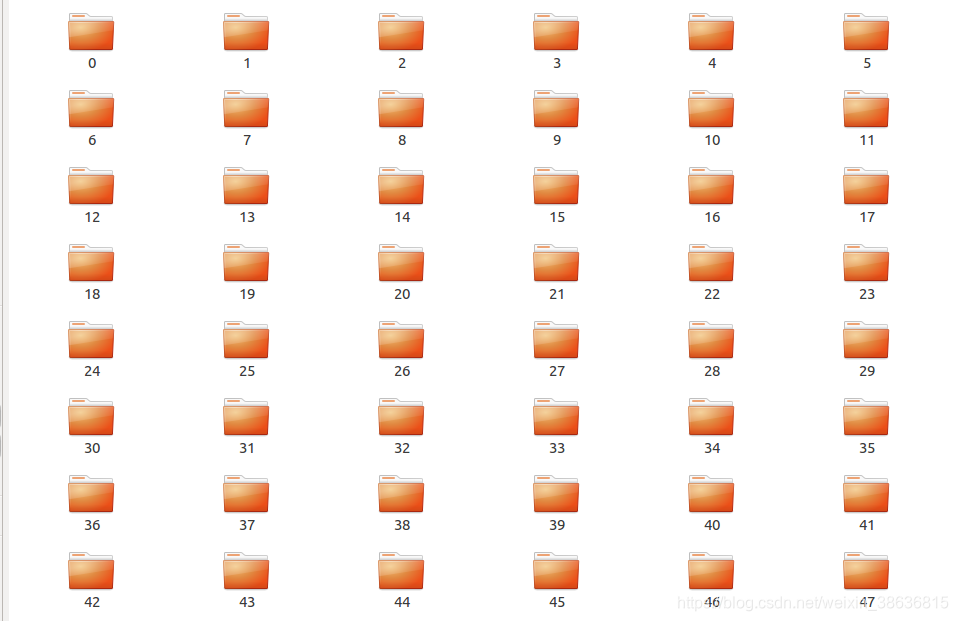
上面的每个文件目录下的文件是这样的.
Bluetooth文件目录
wifi文件目录
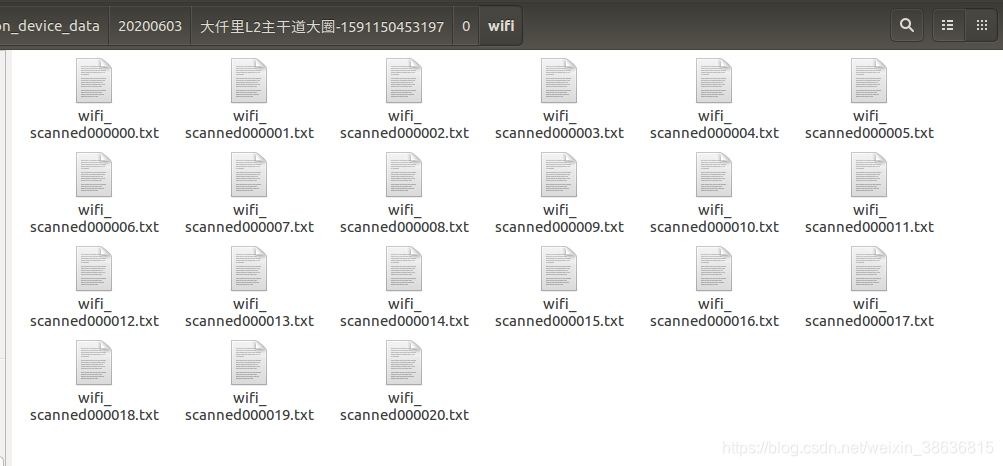
首先是将目录下的所有视频都合并到一起
下面的代码实现视频的合并,文件的合并,多个文件夹下文件的归并
# -*-coding:utf-8-*-
# this python script is to concatenate a sequence of videos into one
# import cv2
from moviepy.editor import *
import os
import linecache
import shutil
#inputVideoPath = "/media/yunlei/Seagate/collection_device_data/20200603/大仟里L2主干道大圈-1591150453197/"
# outputVideoPath = "/media/yunlei/Seagate/collection_device_data/20200603/concatenated/大仟里L2主干道大圈-1591150453197/"
inputVideoPath = "/media/yunlei/Seagate/collection_device_data/20200603/大仟里L2主干道-1591155992285/"
outputVideoPath = "/media/yunlei/Seagate/collection_device_data/20200603/concatenated/大仟里L2主干道-1591155992285/"
def videoconcatenate(left_right_depth):
print("this function is to implement concatenation")
dirs = os.listdir(inputVideoPath)
videoList = []
videoCount = 0
for videoDir in dirs:
videoName = inputVideoPath + str(videoCount) + "/" + left_right_depth + ".mp4"
if os.path.exists(videoName):
videoElement = VideoFileClip(videoName)
videoList.append(videoElement)
videoCount = videoCount + 1
concatenateProcessLeft = concatenate_videoclips(videoList)
concatenateProcessLeft.to_videofile(outputVideoPath + "/" + left_right_depth + ".mp4", fps = 20, remove_temp = False)
def combinefiles(fileName):
print("start to comebine files")
#This list is used to store all file data
filePathList = []
fileDataList = []
fileCount = 0
filePathes = os.listdir(inputVideoPath)
for filePath in filePathes:
fileType = inputVideoPath + str(fileCount) + "/" + fileName + ".txt"
if os.path.exists(fileType):
filePathList.append(fileType)
# print(fileType)
fileCount = fileCount + 1
totalline = 0
for fileElement in filePathList:
lineNumber = 1
fileLength = len(open(fileElement, encoding='utf-8').readlines())
totalline = totalline + fileLength
#print(fileLength)
while lineNumber <= fileLength:
line = linecache.getline(fileElement, lineNumber)
#print(line)
line = line.strip()
fileDataList.append(line)
lineNumber = lineNumber + 1
print(totalline)
fileAll = open(outputVideoPath + "/" + fileName + ".txt", 'w+', encoding='utf-8')
for i, p in enumerate(fileDataList):
print(i,p)
fileAll.write(p+'\n')
fileAll.close()
def combineFolders(folderName):
folderList = []
folderCount = 0
outputFolderPath = outputVideoPath + "/" + folderName + "/"
folderPathes = os.listdir(inputVideoPath)
print(folderPathes)
for folderPath in folderPathes:
folerType = inputVideoPath + "/" + str(folderCount) + "/" + folderName
print("folderType")
print(folerType)
print("start to copy file")
if os.path.exists(folerType):
filesInFolder = os.listdir(folerType)
print("filesInFolder")
print(filesInFolder)
for fileInFolder in filesInFolder:
totalPath = folerType + "/" + fileInFolder
print("print totalPath")
print(totalPath)
if not os.path.exists(outputFolderPath):
os.mkdir(outputFolderPath)
outputFileName = outputFolderPath + "/" + fileInFolder
shutil.copyfile(totalPath, outputFileName)
folderCount = folderCount + 1
#define the main function,from this function your users functions are called
def main():
# combine Bluetooth folder
combineFolders("Bluetooth")
# combine wifi folder
combineFolders("wifi")
# concatenate video left
videoconcatenate("left")
# # # concatenate video right
videoconcatenate("right")
# # #concatenate video depth
videoconcatenate("depth")
# combinefiles("video_time")
combinefiles("Camera_time")
# combinefiles("Bluetooth_times")
combinefiles("wifi_times")
#the entrance of this projrct
if __name__ == "__main__":
main()
因为是刚学习python所以很多时候并不知哪个用法更合适,所以那就尝试一下,比如下面这两个遍历路径下的文件的方式,
for videoDir in dirs:
这种,会将dirs路径下的所有文件都获取到,如果比如说我这里的路径下就包括了文件加和文件,而我希望对文件夹做处理,所以我就要先将文件夹挑拣出来.下面就是我只检索那些是文件夹,并且文件夹上有.mp4格式视频的文件目录我才把他们count in.
videoLeft = inputVideoPath + str(videoCount) + "/" + "left.mp4"
videoRight = inputVideoPath + str(videoCount) + "/" + "right.mp4"
videoDepth = inputVideoPath + str(videoCount) + "/" + "depth.mp4"
if os.path.exists(videoLeft):
第二种,这种os.walk(path)的方式可以返回root就是根目录path,dirs就是root目录下所有的文件夹,以及文件夹下的文件夹,files就是root path下所有的文件.所以你需要根据你的需求来选择使用哪种遍历方式.
for root, dirs, files in os.walk(inputVideoPath):
for name in files:
print(os.path.join(root, name))
for name in dirs:
print(os.path.join(root, name))
print(len(videoLeftAll))
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)