用iris数据进行分类训练,并可视化
首先导入API:
import torch
import torch.nn.functional as F
import matplotlib.pyplot as plt
from sklearn.decomposition import PCA
from torch.autograd import Variable
from sklearn.datasets import load_iris
import pandas as pd
import numpy as np
获得数据集:
iris = load_iris()
iris_d = pd.DataFrame(iris['data'], columns = ['Sepal_Length', 'Sepal_Width', 'Petal_Length', 'Petal_Width'])
iris_d['Species'] = iris.target
特征降维——主成分分析(PCA),将四维降至两维
transfer_1 = PCA(n_components=2)
iris_d = transfer_1.fit_transform(iris_d)
x = torch.from_numpy(iris_d)
y =torch.from_numpy(iris.target)
x, y = Variable(x), Variable(y)
构建前向神经网络,2个输入神经元,10个中间层神经元,3个输出神经元,激活函数使用RELU:
net =torch.nn.Sequential(
torch.nn.Linear(2, 10),
torch.nn.ReLU(),
torch.nn.Linear(10, 3),
)
print(net)
设定autograd为梯度下降法以及损失函数为交叉熵误差:
optimizer = torch.optim.SGD(net.parameters(), lr=0.2) #随机梯度下降
loss_func = torch.nn.CrossEntropyLoss()
训练模型,循环150次:
for t in range(150):
out = net(x.float()) # input x and predict based on x
loss = loss_func(out, y.long()) # must be (1. nn output, 2. target), the target label is NOT one-hotted
optimizer.zero_grad() # clear gradients for next train
loss.backward() # backpropagation, compute gradients
optimizer.step() # apply gradients
可视化,每十步更新一次:
if t % 10 == 0:
# plot and show learning process
plt.cla()
prediction = torch.max(out, 1)[1]
pred_y = prediction.data.numpy()
target_y = y.data.numpy()
plt.scatter(x.data.numpy()[:, 0], x.data.numpy()[:, 1], c=pred_y, s=100, lw=0, cmap='RdYlGn')
accuracy = float((pred_y == target_y).astype(int).sum()) / float(target_y.size)
plt.text(1.5, -4, 'Accuracy=%.2f' % accuracy, fontdict={'size': 20, 'color': 'red'})
plt.pause(0.1)
plt.show()
保存及加载神经网络模型:
torch.save(net, 'E:\\net.pkl') # save entire net
torch.save(net.state_dict(), 'E:\\net_params.pkl') # save only the parameters
net1 = torch.load('net.pkl') #加载神经网络
附完整代码:
import torch
import torch.nn.functional as F
import matplotlib.pyplot as plt
from sklearn.decomposition import PCA
from torch.autograd import Variable
# make fake data
from sklearn.datasets import load_iris
import pandas as pd
import numpy as np
iris = load_iris()
iris_d = pd.DataFrame(iris['data'], columns = ['Sepal_Length', 'Sepal_Width', 'Petal_Length', 'Petal_Width'])
iris_d['Species'] = iris.target
#特征降维——主成分分析
transfer_1 = PCA(n_components=2)
iris_d = transfer_1.fit_transform(iris_d)
x = torch.from_numpy(iris_d)
y =torch.from_numpy(iris.target)
x, y = Variable(x), Variable(y)
# mehod
net =torch.nn.Sequential(
torch.nn.Linear(2, 10),
torch.nn.ReLU(),
torch.nn.Linear(10, 3),
)
#net1 = Net(n_feature=2, n_hidden=10, n_output=3) # define the network
print(net) # net architecture
optimizer = torch.optim.SGD(net.parameters(), lr=0.2) #随机梯度下降
loss_func = torch.nn.CrossEntropyLoss() # the target label is NOT an one-hotted
for t in range(150):
out = net(x.float()) # input x and predict based on x
loss = loss_func(out, y.long()) # must be (1. nn output, 2. target), the target label is NOT one-hotted
optimizer.zero_grad() # clear gradients for next train
loss.backward() # backpropagation, compute gradients
optimizer.step() # apply gradients
if t % 10 == 0:
# plot and show learning process
plt.cla()
prediction = torch.max(out, 1)[1]
pred_y = prediction.data.numpy()
target_y = y.data.numpy()
plt.scatter(x.data.numpy()[:, 0], x.data.numpy()[:, 1], c=pred_y, s=100, lw=0, cmap='RdYlGn')
accuracy = float((pred_y == target_y).astype(int).sum()) / float(target_y.size)
plt.text(1.5, -4, 'Accuracy=%.2f' % accuracy, fontdict={'size': 20, 'color': 'red'})
plt.pause(0.1)
plt.show()
torch.save(net, 'E:\\net.pkl') # save entire net
torch.save(net.state_dict(), 'E:\\net_params.pkl') # save only the parameters
net1 = torch.load('net.pkl') #加载神经网络
训练结果展示:
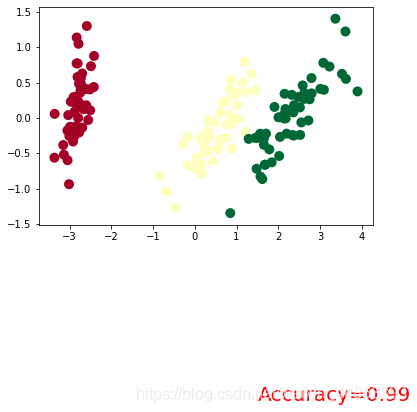