目录(TOC)是Word文档的重要组成部分。它提供了文档内容的概述,并允许您快速导航到所需的部分。您可能会遇到需要以编程方式从Word文档中添加,提取,更新或删除目录的情况。为此,本文将教您如何使用C ++处理Word文件中的目录。
让我们探索以下有关的内容:
- 在Word文档中添加目录
- 从Word文档中提取目录
- 更新Word文档中的目录
- 从Word文档中删除目录
Aspose.Words for C ++ 是本机C ++库,允许您创建,读取,修改和转换Microsoft Word文档。此外,它还支持使用Word文件中的目录。
>>你可以这里下载Aspose.Words for C ++ 最新版测试体验。
在Word文档中添加目录
以下是在Word文档中添加目录的步骤。
- 使用Document 类加载Word文件 。
- 使用先前创建的Document对象创建DocumentBuilder类的实例 。
- 使用DocumentBuilder-> InsertTableOfContents(System :: String开关)方法插入目录。
- 使用Document-> UpdateFields()方法填充目录。
- 使用Document-> Save(System :: String fileName)方法保存Word文档。
下面的示例代码显示了如何使用C ++在Word文档中添加目录。
// Source and output directory paths.
System::String sourceDataDir = u"SourceDirectory\\";
System::String outputDataDir = u"OutputDirectory\\";
// Load the Word file
System::SharedPtrdoc = System::MakeObject(sourceDataDir + u"Sample 5.docx");
// Create an instance of the DocumentBuilder class
System::SharedPtrbuilder = System::MakeObject(doc);
// Insert a table of contents at the beginning of the document.
builder->InsertTableOfContents(u"\\o \"1-3\" \\h \\z \\u");
// The newly inserted table of contents will be initially empty.
// It needs to be populated by updating the fields in the document.
doc->UpdateFields();
// Output file path
System::String outputPath = outputDataDir + u"AddTOC.docx";
// Save the Word file
doc->Save(outputPath);
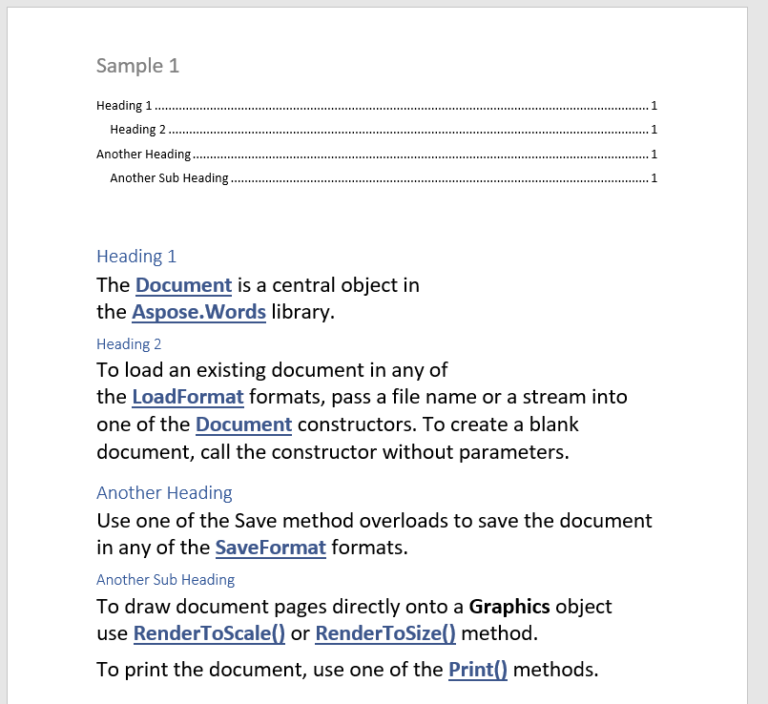
从Word文档中提取目录
以下是从Word文档中提取目录的步骤。
- 使用Document 类加载Word文件 。
- 使用Document-> get_Range()-> get_Fields()方法检索字段并在它们上循环。
- 检查字段是否为FieldType :: FieldHyperlink类型。
- 检查该字段是否属于目录。
- 检索并打印字段信息。
下面的示例代码演示了如何使用C ++从Word文档中提取目录。
// Source direvctory
System::String inputDataDir = u"SourceDirectory\\";
// Load the Word file
System::SharedPtrdoc = System::MakeObject(inputDataDir + u"SampleTOC.docx");
// Loop through the fields
for (System::SharedPtrfield : System::IterateOver(doc->get_Range()->get_Fields()))
{
// Get FieldHyperlink fields
if (field->get_Type() == FieldType::FieldHyperlink)
{
System::SharedPtrhyperlink = System::DynamicCast(field);
// Check if field belongs to TOC
if (hyperlink->get_SubAddress() != nullptr && hyperlink->get_SubAddress().StartsWith(u"_Toc"))
{
System::SharedPtrtocItem = System::DynamicCast(field->get_FieldStart()->GetAncestor(NodeType::Paragraph));
std::cout << System::StaticCast(tocItem)->ToString(SaveFormat::Text).Trim().ToUtf8String() << std::endl; std::cout << "------------------" << std::endl; if (tocItem != nullptr) { System::SharedPtrbm = doc->get_Range()->get_Bookmarks()->idx_get(hyperlink->get_SubAddress());
// Get the location this TOC Item is pointing to
System::SharedPtrpointer = System::DynamicCast(bm->get_BookmarkStart()->GetAncestor(NodeType::Paragraph));
std::cout << System::StaticCast(pointer)->ToString(SaveFormat::Text).ToUtf8String() << std::endl; } } } }
更新Word文档中的目录
如果文档的内容已更新,并且您需要在目录中反映这些更改,则只需加载Word文件并调用 Document-> UpdateFields() 方法。此方法将根据修改后的内容更新目录。之后,保存更新的Word文档。
以下是从Word文档中删除目录的步骤。
- 使用Document 类加载Word文件 。
- 检索并存储FieldStart节点的列表。
- 遍历节点,直到到达指定目录结尾的类型NodeType :: FieldEnd的节点。
- 使用Node-> Remove()方法删除目录。
- 使用Document-> Save(System :: String fileName)方法保存Word文档。
下面的示例代码显示了如何使用C ++从Word文档中删除目录。
void RemoveTableOfContents(const System::SharedPtr& doc, int32_t index)
{
// Store the FieldStart nodes of TOC fields in the document for quick access.
std::vectorfieldStarts;
// This is a list to store the nodes found inside the specified TOC. They will be removed
// at the end of this method.
std::vectornodeList;
for (System::SharedPtrstart : System::IterateOver(doc->GetChildNodes(NodeType::FieldStart, true)))
{
if (start->get_FieldType() == FieldType::FieldTOC)
{
// Add all FieldStarts which are of type FieldTOC.
fieldStarts.push_back(start);
}
}
// Ensure that the TOC specified by the passed index exists.
if (index > fieldStarts.size() - 1)
{
throw System::ArgumentOutOfRangeException(u"TOC index is out of range");
}
bool isRemoving = true;
// Get the FieldStart of the specified TOC.
System::SharedPtrcurrentNode = fieldStarts[index];
while (isRemoving)
{
// It is safer to store these nodes and delete them all at once later.
nodeList.push_back(currentNode);
currentNode = currentNode->NextPreOrder(doc);
// Once we encounter a FieldEnd node of type FieldTOC then we know we are at the end
// of the current TOC and we can stop here.
if (currentNode->get_NodeType() == NodeType::FieldEnd)
{
System::SharedPtrfieldEnd = System::DynamicCast(currentNode);
if (fieldEnd->get_FieldType() == FieldType::FieldTOC)
{
isRemoving = false;
}
}
}
// Remove all nodes found in the specified TOC.
for (System::SharedPtrnode : nodeList)
{
node->Remove();
}
}
int main()
{
// Source and output directory paths.
System::String sourceDataDir = u"SourceDirectory\\";
System::String outputDataDir = u"OutputDirectory\\";
// Open a Word document
System::SharedPtrdoc = System::MakeObject(sourceDataDir + u"SampleTOC.docx");
// Remove the first table of contents from the document.
RemoveTableOfContents(doc, 0);
// Output file path
System::String outputPath = outputDataDir + u"RemoveTOC.docx";
// Save the Word file
doc->Save(outputPath);
}
如果您有任何疑问或需求,请随时加入Aspose技术交流群(761297826),我们很高兴为您提供查询和咨询。