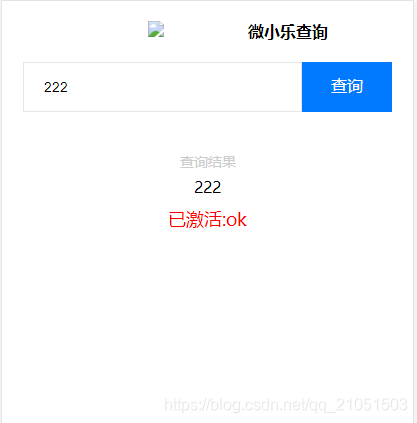
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,maximum-scale=1, minimum-scale=1, user-scalable=no">
<title>Activation code</title>
<style type="text/css">
*{margin: 0;padding: 0}
html,body{width: 100%;height: 100%;}
.img-logo{display: block;margin: 20px auto; width:120px;}
.code-box{margin: 0 5%;position:relative;width: 90%;height:50px;padding: 20px 0;}
.input-code,.check{position:absolute;height: 50px;line-height: 50px;}
.input-code{left:0;width:calc(100% - 90px);border: 1px solid #e6e6e6;outline-style: none;box-sizing: border-box;font-size: 14px;padding-left: 20px}
.check{right: 0;width: 90px;color: #FFF;font-size:16px;background: #017AFF;border: hidden;outline-style: none;}
.tips-box{margin-top: 20px;display: none;}
.tips{width: 100%;text-align: center;}
.tip-title{color: #ccc;font-size: 14px;}
.tip-code{color: #000;font-size: 16px;margin-top: 5px;}
.tip-res{color: #ff0000;font-size: 18px;margin-top: 10px;}
</style>
</head>
<body>
<img class="img-logo" src="logo.png">
<h4 style="text-align: center;">微小乐查询</h4>
<div class="code-box">
<input class="input-code" type="text" placeholder="请输入激活码">
<button class="check">查询</button>
</div>
<div class="tips-box">
<p class="tips tip-title">查询结果</p>
<p class="tips tip-code"></p>
<p class="tips tip-res"></p>
</div>
</body>
<script type="text/javascript" src="http://coolaf.com/static/js/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
function checkCode() {
var code = $('.input-code').val(),
url = './aaaammcheck.php?pin=' + code;
$('.tip-code').html(code)
$.ajax({
url: url,
type: 'GET',
dataType:'JSON',
success: function (data) {
$('.tip-res').html('')
if (data.code == 0){
// var data = d.payload
if (data.status == 0) {
$('.tip-res').html('不存在')
} else if (data.status == 1) {
$('.tip-res').html('未激活')
} else if (data.status == 2) {
$('.tip-res').html('已激活:' + data.register)
} else if (data.status == 3) {
$('.tip-res').html('失效')
}
}else{
$('.tip-res').html(d.err_msg)
}
},
error: function (err) {$('.tip-res').html('服务异常')},
complete: function () {
$('.tips-box').fadeIn()
}
})
}
function init() {
$('.input-code').on('input',function () {
var len = $(this).val().length;
if (len == 0 ) {
$('.tips-box').hide()
}
})
$('.check').on('click',function () {
checkCode()
})
}
init()
})
</script>
</html>
//aaaammcheck.php
<?php
// f (data.status == 0) {
// $('.tip-res').html('不存在')
// } else if (data.status == 1) {
// $('.tip-res').html('未激活')
// } else if (data.status == 2) {
// $('.tip-res').html('已激活:' + data.register)
// } else if (data.status == 3) {
// $('.tip-res').html('失效')
// }
$pin = isset($_REQUEST["pin"]) ? $_REQUEST["pin"] : ""; //
if($pin && $pin != ""){
include "aaaammSql.php";
$sn_rs = $db->select("alipayshouquan", "signkey='$pin'");
if (!empty($sn_rs)) {
$alipayid = isset($sn_rs['alipayid']) ? $sn_rs['alipayid'] : "";
$key = isset($sn_rs['signkey']) ? $sn_rs['signkey'] : "";
$status = isset($sn_rs['status']) ? $sn_rs['status'] : "";
if($alipayid == ""){
$arr['status']=1;
}else{
$arr['status']=2;
$arr['register']="ok";
}
if($status == "3"){
$arr['status']=3;
}
}else{
$arr['status']=0;
}
$arr['code']=0;
echo json_encode($arr);
}
?>
//aaaammSql.php
<?php
// require '360_safe3.php';
/**
*
* mysql 数据库连接模块 sql语言处理类模块
*
* 使用说明 :
* 先填写数据库配置
* 默认加载数据库类,文件中直接引入本模块即可
*
* 默认加载:
* $db=new liu_mysql();
*
* 调用方式 : $db->子程序();
*
*
* 数据库类 :
* liu_mysql (主机,用户,密码,表名);
*
*
* 子程序类 :
* 1.SQL查询程序
* select( 表名 ,条件 ,排序, 反回状态(默认一行), 反回结果(默认全部) ,查询条数(默认全部))
*
* 调用方式 : $db->select($name,$where,$order,$type,$retu,$limit);
*
* 2.SQL更新程序
* update( 表名 ,更新内容 ,条件)
*
* 调用方式 : $db->update($name,$set,$where);
*
* 3.SQL删除程序
* del( 表名 ,删除条件)
*
* 调用方式 : $db->del($name,$where);
*
* 4.SQL添加程序(会返回插入的ID)
* info( 表名 ,字段 ,内容)
*
* 调用方式 : $db->info($name,$title,$val);
*
* 5.SQL执行程序 (不直接调用)
* query( SQL语言)
*
* 调用方式 : $db->query($sql);
*
* 6.SQL转数组程序 (不直接调用)
* arrty( SQL结果 ,数组方式 type 1 全部 2 一行 3 单结果)
*
* 调用方式 : $db->arrty($sql,$type);
*
* 7.关闭mysql连接
* no()
*
* 调用方式 : $db->no();
*/
/*-----数据库配置-----*/
$SQL_host = "127.0.0.1";
$SQL_user = "aaaammSql"; //数据库用户名
$SQL_pass = "aaaammSql"; //数据库密码
$SQL_name = "aaaammSql"; //数据库表名
/*-----默认加载数据库类($db变量名,千万不可更改))-----*/
$db = new liu_mysql($SQL_host, $SQL_user, $SQL_pass, $SQL_name);
/*-----连接数据库类-----*/
class liu_mysql {
var $conn; //初始化数据库
/*--加载类时执行程序--*/
function __construct($SQL_host, $SQL_user, $SQL_pass, $SQL_name) {
//执行连接数据库程序
$this->conn_mysql($SQL_host, $SQL_user, $SQL_pass, $SQL_name);
}
/*--连接数据库程序--*/
function conn_mysql($SQL_host, $SQL_user, $SQL_pass, $SQL_name) {
//连接数据库
$this->conn = @mysql_connect($SQL_host, $SQL_user, $SQL_pass);
//如果连接数据库错误,输出错误信息
if (!$this->conn) {
die('Liuchang Mysql: conn' . mysql_error());
}
//选择数据库表名
$select = @mysql_select_db($SQL_name, $this->conn);
//选择数据库表名出错 输出错误信息
if ($select == false) {
die("Liuchang Mysql: select database($SQL_name)");
}
//选择数据库连接编码
$this->query("set names 'utf8'");
return true;
}
/*--数据库语句执行--*/
function query($sql) {
//开始发送查询SET
$res = mysql_query($sql, $this->conn);
//输出错误信息
if (!$res) {
die('Liuchang Mysql: query ' . mysql_error());
}
//返回结果
return $res;
}
/*--数据库结果转数组 type 1 全部 2 一行 3 单结果--*/
function arrty($sql, $type = 1) {
//反回全部数组
if ($type == 1) {
$arr = array();
//循环取结果,加到arr数组
while ($row = mysql_fetch_assoc($sql)) {
$arr[] = $row;
}
//反回arr全部数组
return $arr;
}
//反回一行数组
if ($type == 2) {
//转化成数组
$arr = mysql_fetch_array($sql, MYSQL_ASSOC);
//反回arr一行数组
return $arr;
}
//反回单个数组
if ($type == 3) {
//转化成数组 MYSQL_NUM 已数字为索引3
$arr = mysql_fetch_array($sql, MYSQL_NUM);
//反回arr单个
return $arr[0];
}
}
/*--SELECT SQL查询程序 表名 条件 排序 反回状态(默认一行) 反回结果(默认全部) 查询条数(默认全部)--*/
function select($name, $where = "", $order = "", $type = "2", $retu = "*", $limit = "") {
if (empty($retu)) {
$retu = "*";
}
//组合条件
if ($where != "") {
$where = "where " . $where . " ";
}
//组合排序
if ($order != "") {
$order = "order by " . $order . " ";
}
//组合条数
if ($limit != "") {
$limit = "LIMIT " . $limit . " ";
}
//echo "select $retu from $name $where $order $limit";
//exit;
//执行sql
$res = $this->query("select $retu from $name $where $order $limit");
//组合数组
if ($res) {
$res = $this->arrty($res, $type);
return $res;
} else {
return false;
}
}
/*--UPDATE SQL更新程序 表名 更新内容 条件--*/
function update($name, $set, $where = "") {
//组合条件
if ($where != "") {
$where = "where " . $where;
}
//执行sql
$res = $this->query("UPDATE $name SET $set $where");
return $res;
}
/*--DELETE SQL删除程序 表名 删除条件--*/
function del($name, $where) {
//组合删除条件
$where = "where " . $where;
//执行sql
$res = $this->query("DELETE FROM $name $where");
return $res;
}
/*--INSERT SQL添加程序 表名 字段 内容--*/
function info($name, $title, $val) {
//执行sql
$res = $this->query("INSERT INTO $name($title) VALUES ($val)");
//返回插入ID
$res = mysql_insert_id($this->conn);
return $res;
}
/*--关闭MYSQL连接--*/
function no() {
mysql_close($this->conn);
}
}
?>
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)