功能:
接收串口数据,将串口数据上报到贝壳物联的数据接口
此处为接收0和1数据,上报到贝壳物联
贝壳物联平台通讯协议
ArduinoJson解析
ArduinoJson Assistant非常好用的工具
#include <ESP8266WiFi.h>
#include <ArduinoJson.h>
#include <Ticker.h>
#include <WiFiClient.h>
const char* ssid = "xxxxx";
const char* password = "xxxxxx";
const char* device_id = "xxxxx";
const char* device_key = "xxxxxxx";
const char* interface_id = "xxxxxx";
String heart_string;
const char* host = "www.bigiot.net";
const int port = 8282;
WiFiClient client;
#define bufferSize 8192
uint8_t buf1[bufferSize];
uint16_t i1=0;
uint8_t buf2[bufferSize];
uint16_t i2=0;
bool tcp_ok = 0;
#define packTimeout 5
void WiFi_Connect()
{
Serial.begin(9600);
Serial.println();
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void Heart_Task()
{
StaticJsonDocument<96> heart_json;
heart_json["M"] = "checkin";
heart_json["ID"] = device_id;
heart_json["K"] = device_key;
serializeJson(heart_json, heart_string);
}
void setup() {
WiFi_Connect();
Heart_Task();
}
void loop() {
if(!client.connected()) {
if (!client.connect(host, port)) {
Serial.println("connection failed");
delay(5000);
tcp_ok = 0;
return;
}
}
if(tcp_ok == 0)
{
tcp_ok = 1;
client.println(heart_string);
}
if(client.available()) {
while(client.available()) {
buf1[i1] = (uint8_t)client.read();
if(i1<bufferSize-1) i1++;
}
StaticJsonDocument<128> receive_json;
DeserializationError error = deserializeJson(receive_json, buf1, i1);
if (error) {
Serial.print(F("deserializeJson() failed: "));
Serial.println(error.f_str());
return;
}
serializeJson(receive_json, Serial);
const char* M = receive_json["M"];
if(*M == 'b')
{
Serial.println(" receive heart beat");
client.println(heart_string);
}
i1 = 0;
}
if(Serial.available()) {
while(1) {
if(Serial.available()) {
buf2[i2] = (char)Serial.read();
if(i2<bufferSize-1) i2++;
} else {
delay(packTimeout);
if(!Serial.available()) {
break;
}
}
}
Serial.write(buf2, i2);
bool send_flag = 0;
StaticJsonDocument<96> send_json;
if(buf2[0] == '0')
{
send_flag = 1;
send_json["V"][interface_id] = "0";
}
else if(buf2[0] == '1')
{
send_flag = 1;
send_json["V"][interface_id] = "1";
}
if(send_flag == 1)
{
String send_string;
send_json["M"] = "update";
send_json["ID"] = device_id;
serializeJson(send_json, send_string);
client.println(send_string);
}
i2 = 0;
send_flag = 0;
}
}
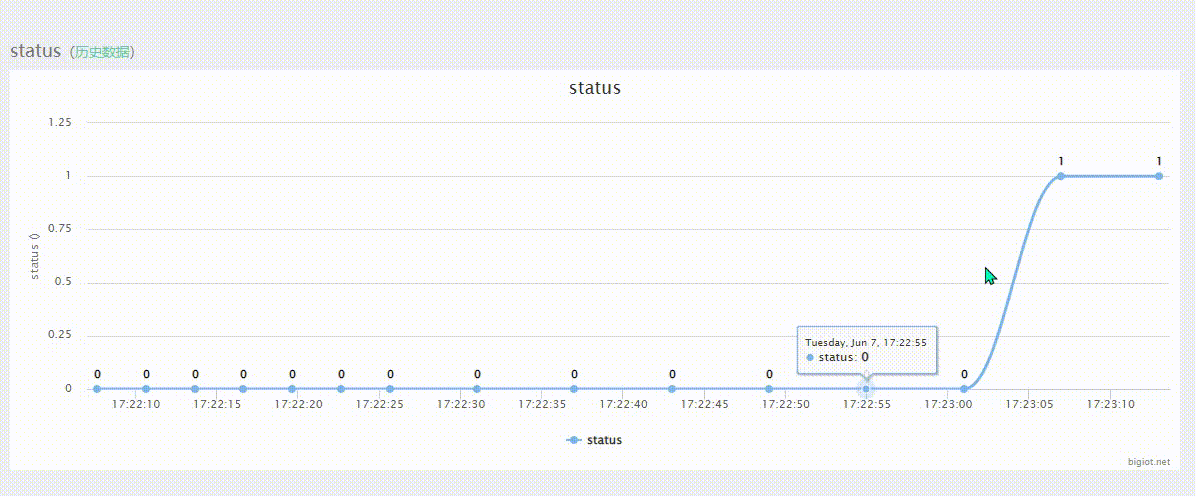
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)