UE4 C++ 一个Character踩地雷
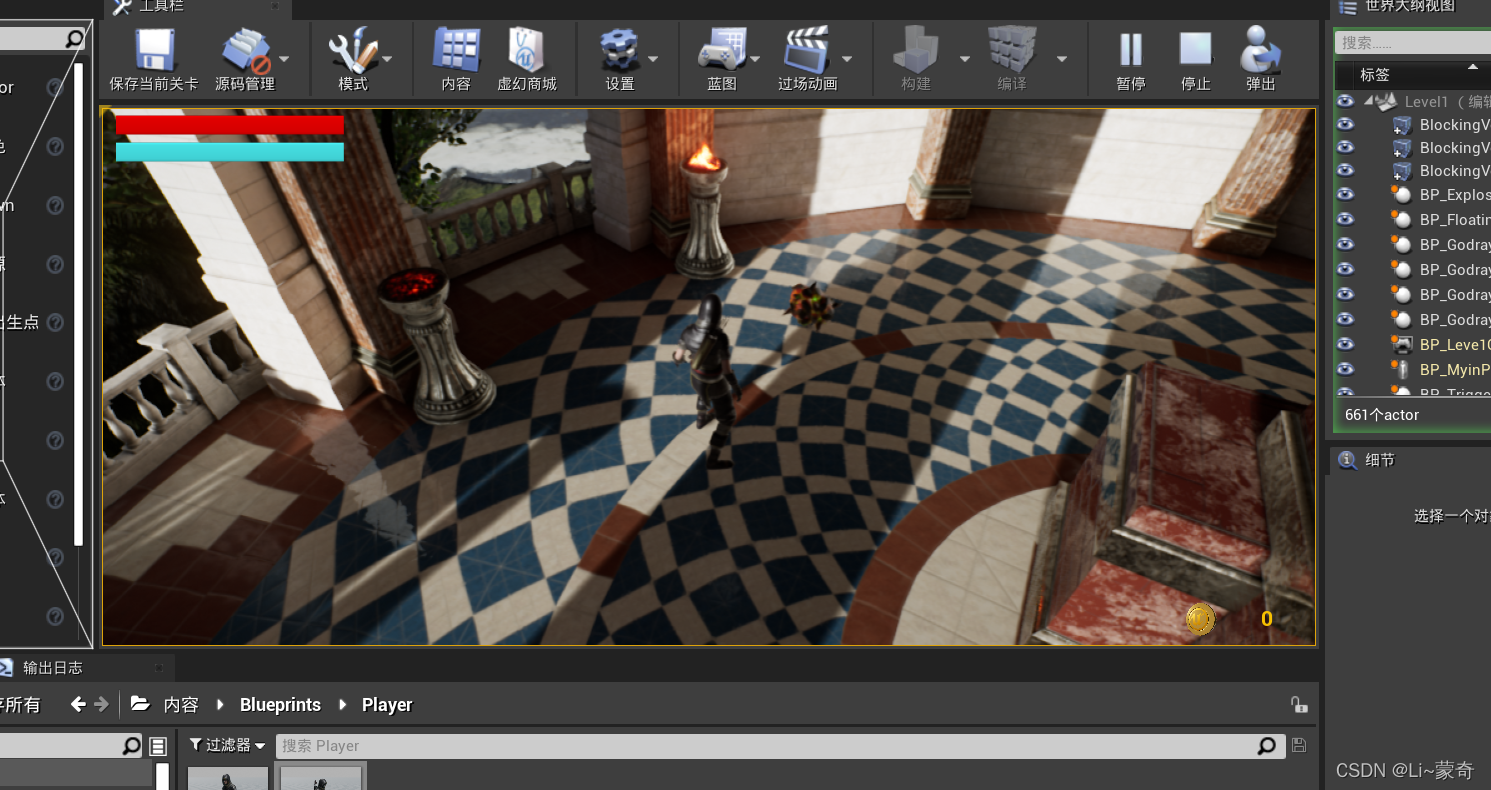
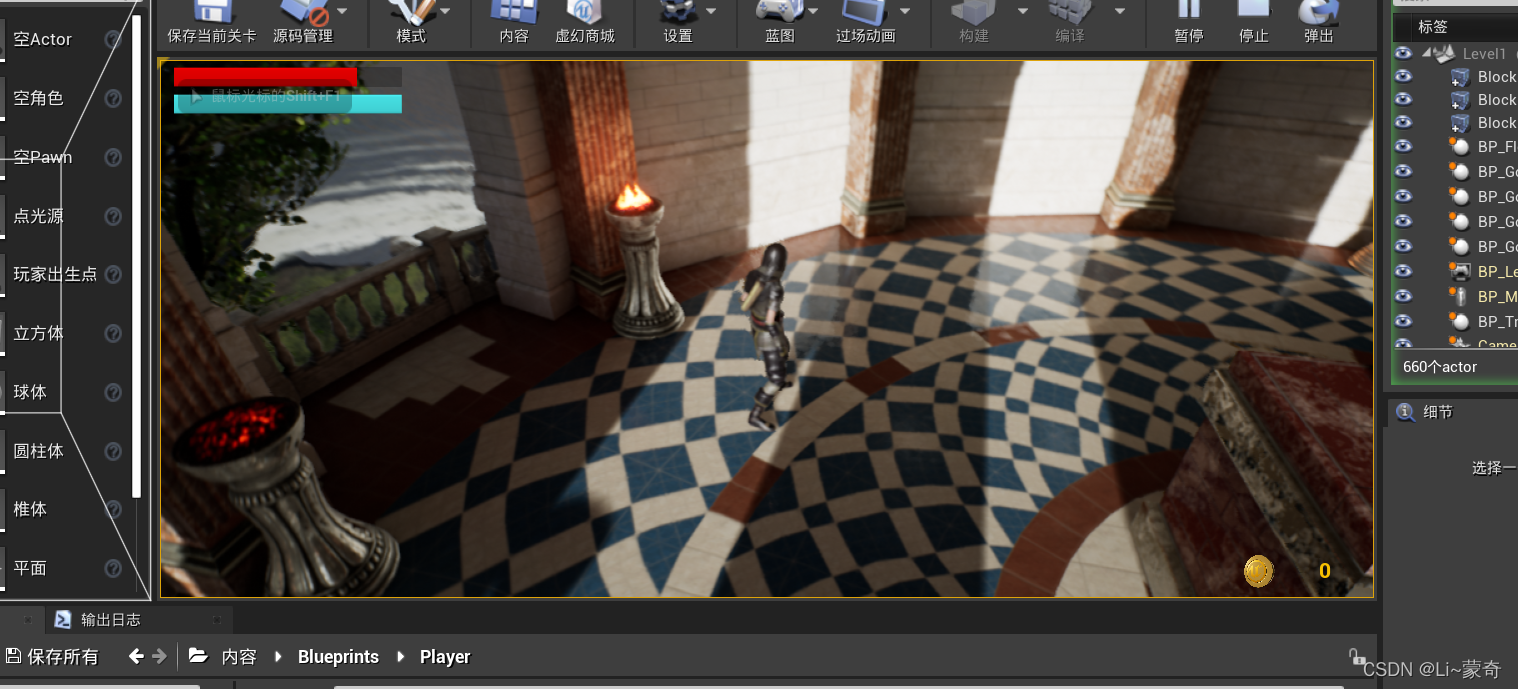
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Character.h"
#include "MyinPlayer.generated.h"
UCLASS()
class GETSTARTED_API AMyinPlayer : public ACharacter
{
GENERATED_BODY()
public:
// Sets default values for this character's properties
AMyinPlayer();
UPROPERTY(VisibleAnywhere,BlueprintReadOnly)
class USpringArmComponent* SpringArm;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly)
class UCameraComponent* FollowCamera;
float BaseTurnRate;
float BaseTurnUpRate;
UPROPERTY(EditDefaultsOnly, BlueprintReadOnly, Category = "Player Stats")
float MaxHealth;//生命值
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Player Stats")
float Health;
UPROPERTY(EditDefaultsOnly, BlueprintReadOnly, Category = "Player Stats")
float MaxStamina;//耐力
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Player Stats")
float Stamina;
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Player Stats")
int32 Coins;
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
// Called to bind functionality to input
virtual void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
virtual void Jump() override;//重写的一个函数
void MoveForward(float value);
void MoveRight(float value);
void Turn(float value);
void LookUp(float value);
void TurnAtRate(float value);
void LookUpAtRate(float value);
void IncreaseHealth(float value);
void IncreaseStamina(float value);
void IncreaseCoin(int value);
virtual float TakeDamage(float Damage, struct FDamageEvent const& DamageEvent, AController* EventInstigator, AActor* DamageCauser) override;
};
这里就是给角色加了一个事件任意伤害
// Fill out your copyright notice in the Description page of Project Settings.
#include "Characters/Player/MyinPlayer.h"
#include "GameFramework/SpringArmComponent.h"
#include "Camera/CameraComponent.h"
#include "Components/CapsuleComponent.h"
#include "Components/InputComponent.h"
#include "GameFramework/CharacterMovementComponent.h"
// Sets default values
AMyinPlayer::AMyinPlayer()
{
// Set this character to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
SpringArm=CreateDefaultSubobject<USpringArmComponent>(TEXT("SpringArm"));
SpringArm->SetupAttachment(GetRootComponent());
SpringArm->TargetArmLength = 600.0f;
SpringArm->bUsePawnControlRotation = true;
FollowCamera=CreateDefaultSubobject<UCameraComponent>(TEXT("FollowCamera"));
FollowCamera->SetupAttachment(SpringArm, USpringArmComponent::SocketName);
FollowCamera->bUsePawnControlRotation = false;
GetCapsuleComponent()->SetCapsuleSize(25.0f, 100.0f);//设置胶囊体组件的高和宽
bUseControllerRotationYaw = false;
GetCharacterMovement()->bOrientRotationToMovement = true;//继承角色移动类里面的“将旋转朝向远动”设置为
GetCharacterMovement()->RotationRate=FRotator(0.0f, 500.0f, 0.0f);//继上面“旋转速率”
BaseTurnRate = 65.0f;
BaseTurnUpRate = 65.0f;
GetCharacterMovement()->JumpZVelocity = 500.0f;//跳的时候一个速度Z轴的速度
GetCharacterMovement()->AirControl = 0.15f;//这是在空中的移动速度
MaxHealth = 100.0f;
Health = MaxHealth;
MaxStamina = 150.f;
Stamina = MaxStamina;
Coins = 0;
}
// Called when the game starts or when spawned
void AMyinPlayer::BeginPlay()
{
Super::BeginPlay();
}
// Called every frame
void AMyinPlayer::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
// Called to bind functionality to input
void AMyinPlayer::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
check(PlayerInputComponent);//是否有效,可用性的检查
PlayerInputComponent->BindAxis("MoveForward", this, &AMyinPlayer::MoveForward);//第一个是轴映射的名称
PlayerInputComponent->BindAxis("MoveRight", this, &AMyinPlayer::MoveRight);
PlayerInputComponent->BindAxis("Turn", this, &AMyinPlayer::Turn);
PlayerInputComponent->BindAxis("LookUp", this, &AMyinPlayer::LookUp);
PlayerInputComponent->BindAxis("TurnAtRate", this, &AMyinPlayer::TurnAtRate);
PlayerInputComponent->BindAxis("LookUpAtRate", this, &AMyinPlayer::LookUpAtRate);//轴
PlayerInputComponent->BindAction("Jump", IE_Pressed, this, &AMyinPlayer::Jump);//按键
PlayerInputComponent->BindAction("Jump", IE_Released, this, &AMyinPlayer::StopJumping);//落下
}
void AMyinPlayer::Jump()//跳跃
{
Super::Jump();
}
void AMyinPlayer::MoveForward(float value)
{
//if ((Controller != nullptr) && (value != 0.0f)) {
//FRotator Rotation = Controller->GetControlRotation();
//FRotator YawRotation(0.0f, Rotation.Yaw, 0.0f);
//FVector Direction = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::X);
//AddMovementInput(Direction, value);
//}
AddMovementInput(FollowCamera->GetForwardVector(), value);//添加移动输入
}
void AMyinPlayer::MoveRight(float value)
{
//if ((Controller != nullptr) && (value != 0.0f)) {
//FRotator Rotation = Controller->GetControlRotation();
//FRotator YawRotation(0.0f, Rotation.Yaw, 0.0f);
//FVector Direction = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::Y);
//AddMovementInput(Direction, value);
//}
AddMovementInput(FollowCamera->GetRightVector(), value);
}
void AMyinPlayer::Turn(float value)
{
if (value != 0.0f)
{
AddControllerYawInput(value);
}
}
void AMyinPlayer::LookUp(float value)
{
if (GetControlRotation().Pitch < 270.0f && GetControlRotation().Pitch>180.0f && value > 0.0f)
{
return;
}
if (GetControlRotation().Pitch > 45.0f && GetControlRotation().Pitch < 180.0f && value < 0.0f) {
return;
}
AddControllerPitchInput(value);
}
void AMyinPlayer::TurnAtRate(float value)
{
float valuee = value * BaseTurnRate*GetWorld()->GetDeltaSeconds();//乘以一个Time函数里的DeltaTime,作用120帧和30帧的电脑跑起来一样快
if (valuee != 0.0f)
{
AddControllerYawInput(value);
}
}
void AMyinPlayer::LookUpAtRate(float value)
{
float valuee = value * BaseTurnUpRate * GetWorld()->GetDeltaSeconds();//乘以一个Time函数里的DeltaTime,作用120帧和30帧的电脑跑起来一样快
if (GetControlRotation().Pitch < 270.0f && GetControlRotation().Pitch>180.0f && value > 0.0f)
{
return;
}
if (GetControlRotation().Pitch > 45.0f && GetControlRotation().Pitch < 180.0f && value < 0.0f) {
return;
}
AddControllerPitchInput(valuee);
}
void AMyinPlayer::IncreaseStamina(float value)
{
Health = FMath::Clamp(Health + value, 0.0f, MaxHealth);
}
void AMyinPlayer::IncreaseCoin(int value)
{
Stamina = FMath::Clamp(Stamina + value, 0.0f, MaxStamina);
}
//事件任意伤害
float AMyinPlayer::TakeDamage(float Damage, FDamageEvent const& DamageEvent, AController* EventInstigator, AActor* DamageCauser)
{
if (Health - Damage <= 0.0f) {
Health = FMath::Clamp(Health - Damage, 0.0f, MaxHealth);
}
else {
Health -= Damage;
}
return Health;
}
void AMyinPlayer::IncreaseHealth(float value)
{
Coins += value;
}
炸弹:
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Gameplay/Interactableitem.h"
#include "Explosiveitem.generated.h"
/**
*
*/
UCLASS()
class GETSTARTED_API AExplosiveitem : public AInteractableitem
{
GENERATED_BODY()
public :
AExplosiveitem();
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Damage")
float Damage;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Damage")
TSubclassOf<UDamageType> DamageTypeClass;
public :
virtual void OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult) override;
virtual void OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex) override;
};
// Fill out your copyright notice in the Description page of Project Settings.
#include "Gameplay/Explosiveitem.h"
#include "Characters/Player/MyinPlayer.h"
#include "Kismet/GameplayStatics.h"
#include "Sound/SoundCue.h"
#include "Components/SphereComponent.h"
AExplosiveitem::AExplosiveitem() {
Damage = 20.0f;
TriggerVolume->SetSphereRadius(50.0f);
}
void AExplosiveitem::OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
Super::OnOverlapBegin(OverlappedComponent, OtherActor, OtherComp, OtherBodyIndex, bFromSweep, SweepResult);
if (OtherActor) {
const AMyinPlayer* MainPlayer = Cast<AMyinPlayer>(OtherActor);
if (MainPlayer) {
if (OverlapParticle) {
UGameplayStatics::SpawnEmitterAtLocation(this, OverlapParticle, GetActorLocation(), FRotator(0.0f),true);//生成爆炸的位置
}
if (OverlapSound) {
UGameplayStatics::PlaySound2D(this,OverlapSound);//播放声音
}
UGameplayStatics::ApplyDamage(OtherActor,Damage,nullptr,this,DamageTypeClass);//这个就是应用伤害
Destroy();//销毁自己
}
}
}
void AExplosiveitem::OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex)
{
Super::OnOverlapEnd(OverlappedComponent, OtherActor, OtherComp, OtherBodyIndex);
}
炸弹继承这个类:
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "Interactableitem.generated.h"
UCLASS()
class GETSTARTED_API AInteractableitem : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
AInteractableitem();
UPROPERTY(VisibleAnywhere, BlueprintReadWrite)
class USphereComponent* TriggerVolume;
UPROPERTY(VisibleAnywhere, BlueprintReadWrite)
class UStaticMeshComponent* DisplayMesh;
UPROPERTY(VisibleAnywhere, BlueprintReadWrite)
class UParticleSystemComponent* IdleParticleComponent;//粒子系统的组件
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interactable Item|Particles")
class UParticleSystem* OverlapParticle;//重叠播放的粒子,可以看做一个资源
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interactable Item|Sounds")
class USoundCue* OverlapSound;//一个声音的资源吧
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interactable Item|Item Properties")
bool bNeedRotate;//需要旋转吗
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interactable Item|Item Properties")
float RotationRate;//旋转的速率
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
UFUNCTION()
virtual void OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult);
UFUNCTION()
virtual void OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex);
};
// Fill out your copyright notice in the Description page of Project Settings.
#include "Gameplay/Interactableitem.h"
#include "Components/SphereComponent.h"
#include "Components/StaticMeshComponent.h"
#include "particles/ParticleSystemComponent.h"
// Sets default values
AInteractableitem::AInteractableitem()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
TriggerVolume = CreateDefaultSubobject<USphereComponent>(TEXT("TriggerVolume"));
RootComponent = TriggerVolume;
// 组件 ->SetCollisionEnabled(ECollisionEnabled::NoCollision); 注释:没有碰撞
// 组件 ->SetCollisionEnabled(ECollisionEnabled::PhysicsOnly); 注释:只有物理
// 组件 ->SetCollisionEnabled(ECollisionEnabled::QueryAndPhysics); 注释:查询和物理
// 组件 ->SetCollisionEnabled(ECollisionEnabled::QueryOnly); 注释:只有查询
// 组件 ->SetCollisionEnabled(ECollisionEnabled::Type); 注释:目前理解为自定义
TriggerVolume->SetCollisionEnabled(ECollisionEnabled::QueryOnly);//仅查询
TriggerVolume->SetCollisionObjectType(ECollisionChannel::ECC_WorldStatic);//设为世界静态
TriggerVolume->SetCollisionResponseToAllChannels(ECollisionResponse::ECR_Ignore);//让所有频道都忽略
TriggerVolume->SetCollisionResponseToChannel(ECollisionChannel::ECC_Pawn, ECollisionResponse::ECR_Overlap);//再单独开启一个Pawn 频道的一个事件
DisplayMesh = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("DisplayMesh"));
DisplayMesh->SetupAttachment(GetRootComponent());
IdleParticleComponent = CreateDefaultSubobject<UParticleSystemComponent>(TEXT("IdleParticleComponent"));
IdleParticleComponent->SetupAttachment(GetRootComponent());
bNeedRotate = true;
RotationRate = 45.0f;
}
// Called when the game starts or when spawned
void AInteractableitem::BeginPlay()
{
Super::BeginPlay();
TriggerVolume->OnComponentBeginOverlap.AddDynamic(this,&AInteractableitem::OnOverlapBegin);
TriggerVolume->OnComponentEndOverlap.AddDynamic(this, &AInteractableitem::OnOverlapEnd);
}
// Called every frame
void AInteractableitem::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
if (bNeedRotate) {
FRotator NewRotation = GetActorRotation();
NewRotation.Yaw += RotationRate * DeltaTime;
SetActorRotation(NewRotation);
}
}
void AInteractableitem::OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
}
void AInteractableitem::OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex)
{
}
血条绑定就是简单的蓝图绑定了!!!!