Python基础–16(面向对象)
面向对象的三大特征
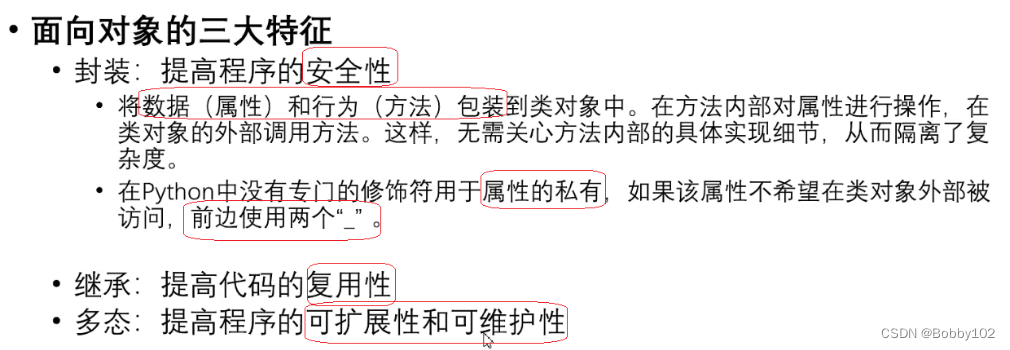
封装
封装的实现
class Student:
def __init__(self,name,age):
self.name=name
self.__age=age
def show(self):
print(self.name,self.__age)
stu=Student('张三',20)
stu.show()
#在类的外部使用name和age
print(stu.name)
#print(stu.__age) print(stu.__age)AttributeError: 'Student' object has no attribute 'name'
print(dir(stu))
print(stu._Student__age)#在类的外部强制访问
#20
继承
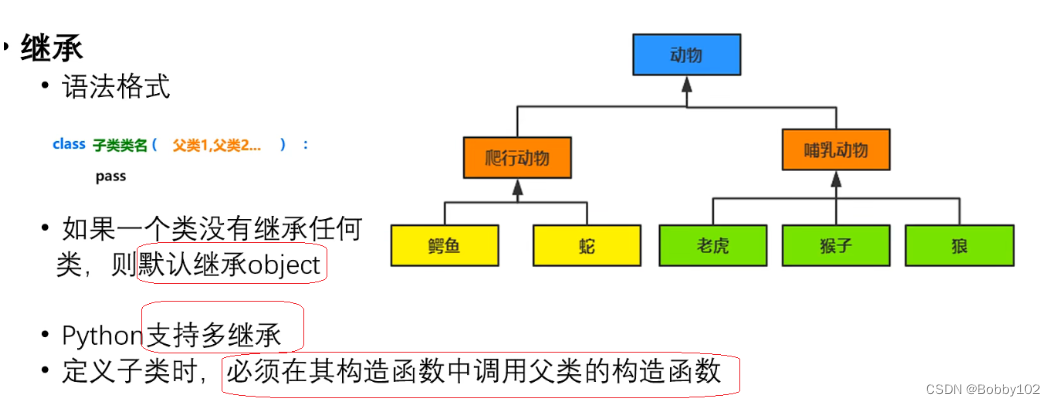
class Person(object):
def __init__(self,name,age):
self.name=name
self.age=age
def info(self):
print('姓名:{0},年龄:{1}'.format(self.name,self.age))
#定义子类
class Student(Person):
def __init__(self,name,age,score):
super().__init__(name,age)
self.score=score
#测试
stu=Student('Jack',20,'1001')
stu.info()
# 姓名:Jack,年龄:20
方法重写
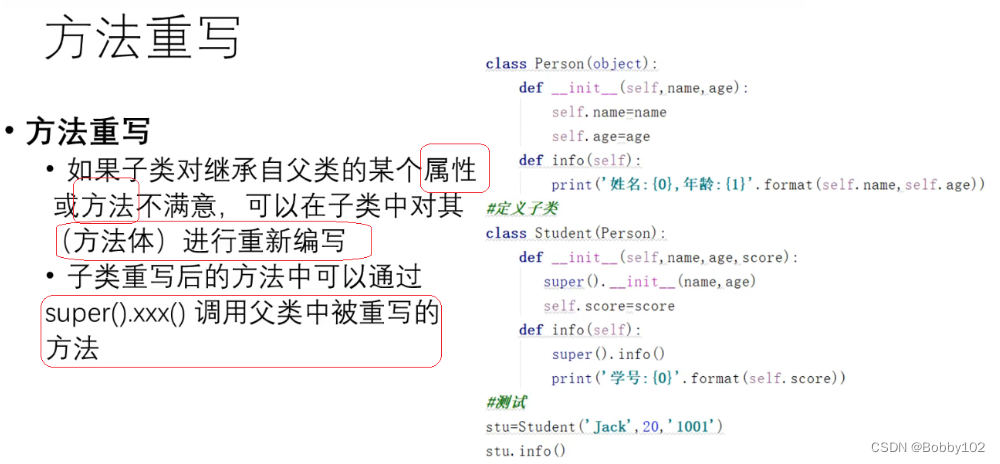
class Person(object):
def __init__(self, name, age):
self.name = name
self.age = age
def info(self):
print(self.name, self.age)
class Student(Person):
def __init__(self, name, age, stu_no):
super().__init__(name, age)
self.stu_no = stu_no
def info(self):
super().info()
print(self.stu_no)
class Teacher(Person):
def __init__(self, name, age, teachofyear):
super().__init__(name, age)
self.teachofyear = teachofyear
stu = Student('张三', 20, '10010')
stu.info()
# 张三 20
# 10010
object类
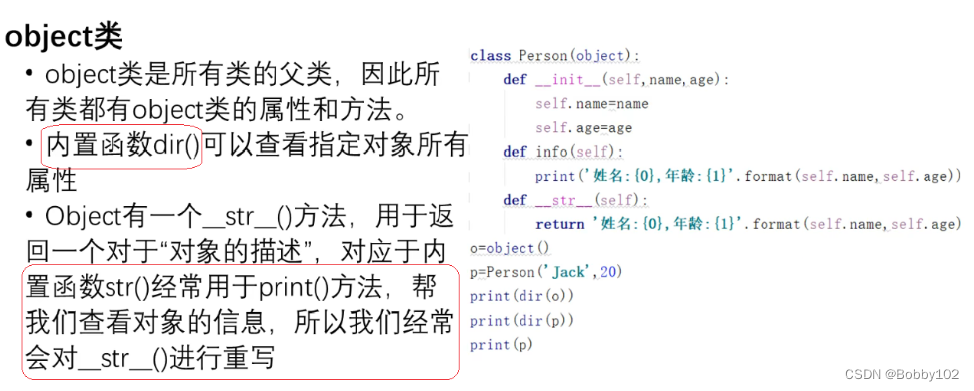
class Student:
def __init__(self,name,age):
self.name=name
self.age=age
def __str__(self):
return '我的名字是{0},今年{1}岁了'.format(self.name,self.age)
stu=Student('张三',20)
print(dir(stu))
# ['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__',
# '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__',
# '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__',
# '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__']
print(stu)
# <__main__.Student object at 0x0000016E05C38400>
# 我的名字是张三,今年20岁了 #默认调用__str__()这样的方法,重写了
多态
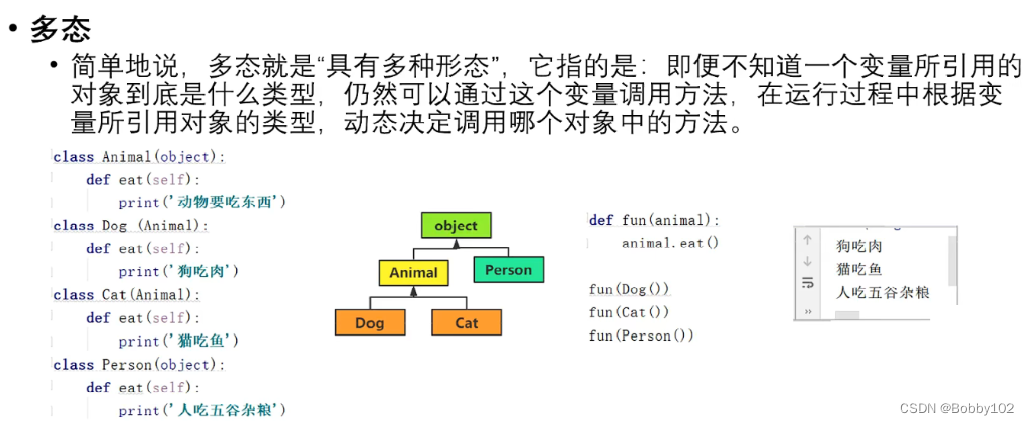
静态语言与动态语言
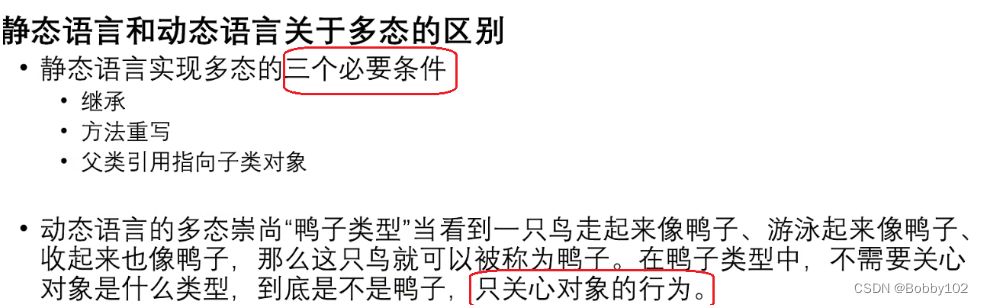
Python是一门动态语言
class Animal(object):
def eat(self):
print('动物吃')
class Dog(Animal):
def eat(self):
print('狗吃')
class Cat(Animal):
def eat(self):
print('猫吃')
class Person(object):
def eat(self):
print('人吃')
def fun(animal):
animal.eat()
fun(Dog())
fun(Cat())
fun(Person())
# 狗吃
# 猫吃
# 人吃