64. Minimum Path Sum
Given a m x n grid filled with non-negative numbers, find a path from top left to bottom right which minimizes the sum of all numbers along its path.
Note: You can only move either down or right at any point in time.
Example:
Input:
[
[1,3,1],
[1,5,1],
[4,2,1]
]
Output: 7
Explanation: Because the path 1→3→1→1→1 minimizes the sum.
solution
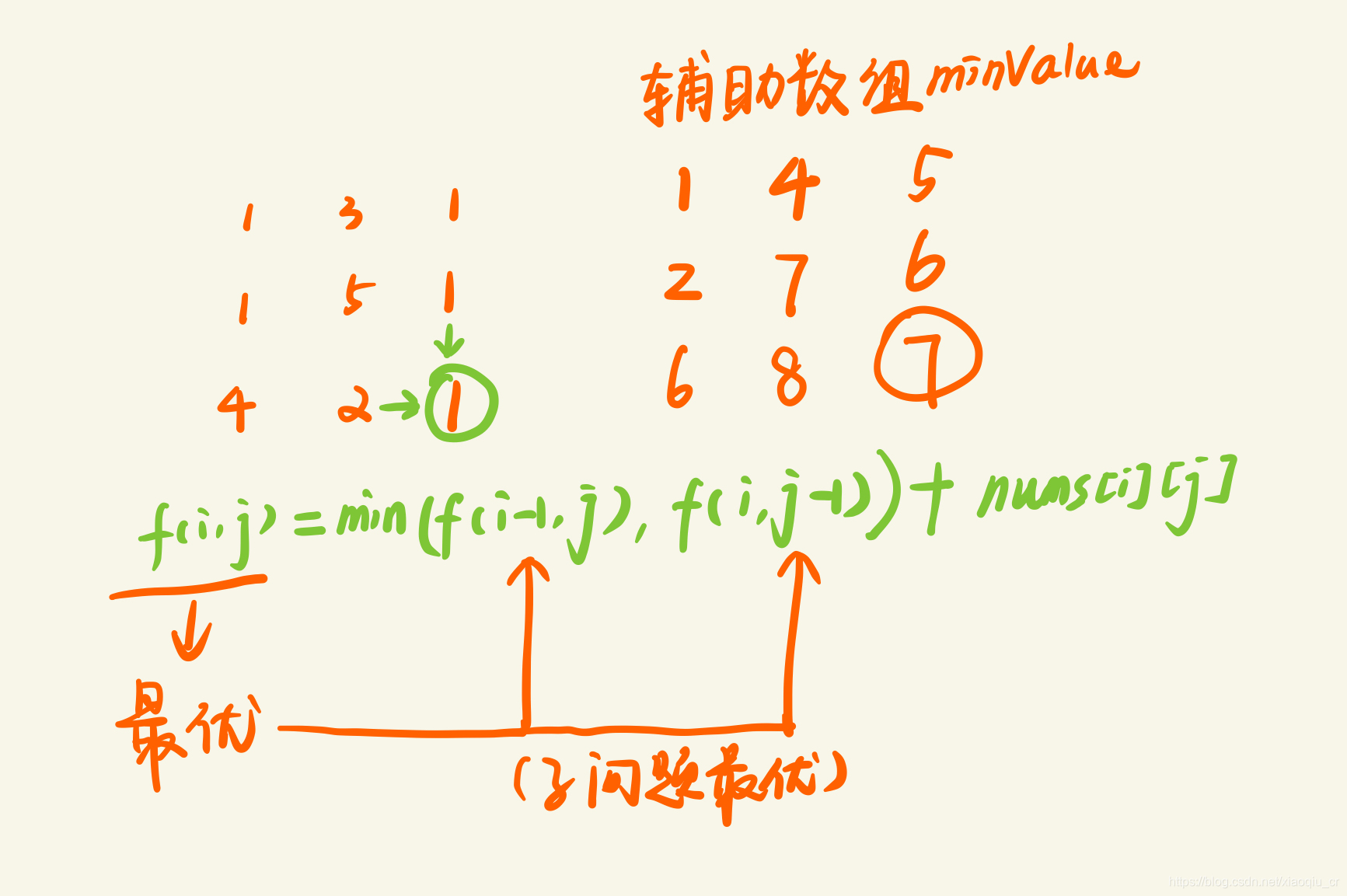
class Solution {
public int minPathSum(int[][] grid) {
int rows = grid.length;
int cols = grid[0].length;
int[][] minValue = new int[rows][cols];
minValue[0][0] = grid[0][0];
for(int i = 1; i < rows; i++){
minValue[i][0] = minValue[i-1][0] + grid[i][0];
}
for(int j = 1; j < cols; j++){
minValue[0][j] = minValue[0][j-1] + grid[0][j];
}
for(int i = 1; i < rows; i ++){
for(int j = 1; j < cols; j++){
minValue[i][j] = Math.min(minValue[i-1][j] , minValue[i][j-1] ) + grid[i][j];
}
}
return minValue[rows-1][cols-1];
}
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)