10. Regular Expression Matching
Given an input string (s
) and a pattern (p
), implement regular expression matching with support for '.'
and '*'
.
'.' Matches any single character.
'*' Matches zero or more of the preceding element.
The matching should cover the entire input string (not partial).
Note:
s
could be empty and contains only lowercase letters a-z
.p
could be empty and contains only lowercase letters a-z
, and characters like .
or *
.
Example 1:
Input:
s = "aa"
p = "a"
Output: false
Explanation: "a" does not match the entire string "aa".
Example 2:
Input:
s = "aa"
p = "a*"
Output: true
Explanation: '*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa".
Example 3:
Input:
s = "ab"
p = ".*"
Output: true
Explanation: ".*" means "zero or more (*) of any character (.)".
Example 4:
Input:
s = "aab"
p = "c*a*b"
Output: true
Explanation: c can be repeated 0 times, a can be repeated 1 time. Therefore, it matches "aab".
Example 5:
Input:
s = "mississippi"
p = "mis*is*p*."
Output: false
solution
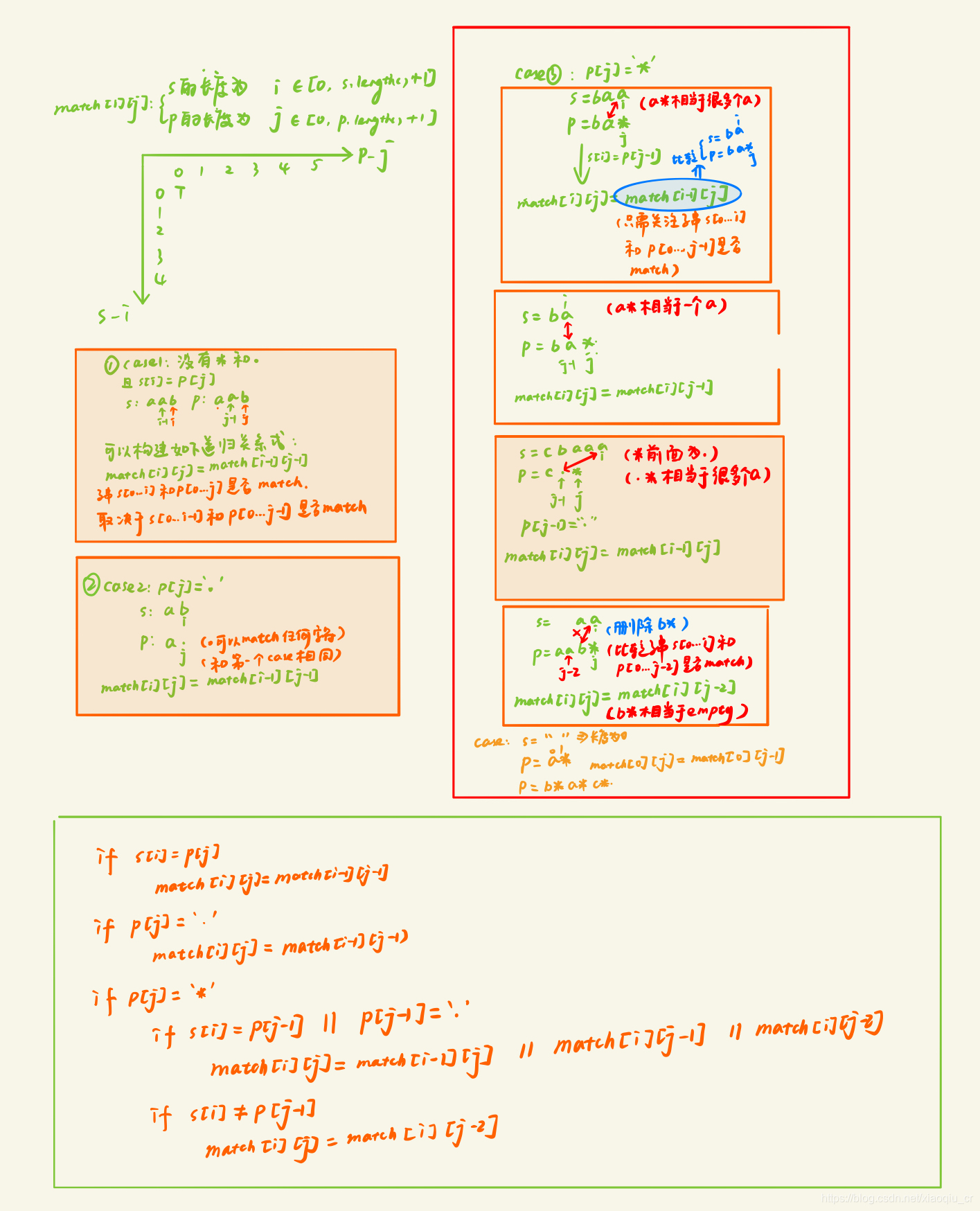
class Solution {
public boolean isMatch(String s, String p) {
if(s == null || p == null) return false;
boolean[][] match = new boolean[s.length() + 1][p.length() + 1];
match[0][0] = true;
for(int j = 1; j <= p.length(); j++){
if(p.charAt(j - 1) == '*' && match[0][j-2]){
match[0][j] = true;
}
}
for(int i = 1; i <= s.length(); i++){
for(int j = 1; j <= p.length(); j++){
if(s.charAt(i-1) == p.charAt(j-1) || p.charAt(j-1) == '.' ){
match[i][j] = match[i-1][j-1];
}else if(p.charAt(j-1) == '*'){
if(s.charAt(i-1) == p.charAt(j-2) || p.charAt(j-2) == '.'){
match[i][j] = match[i-1][j] || match[i][j-2] || match[i][j-1];
}else{
match[i][j] = match[i][j-2];
}
}
}
}
return match[s.length()][p.length()];
}
}
solution2
参考我的另一篇博客:正则表达式匹配
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)