在做一些后台管理会用到很多的表单,比如动态项表单,如下图这样的
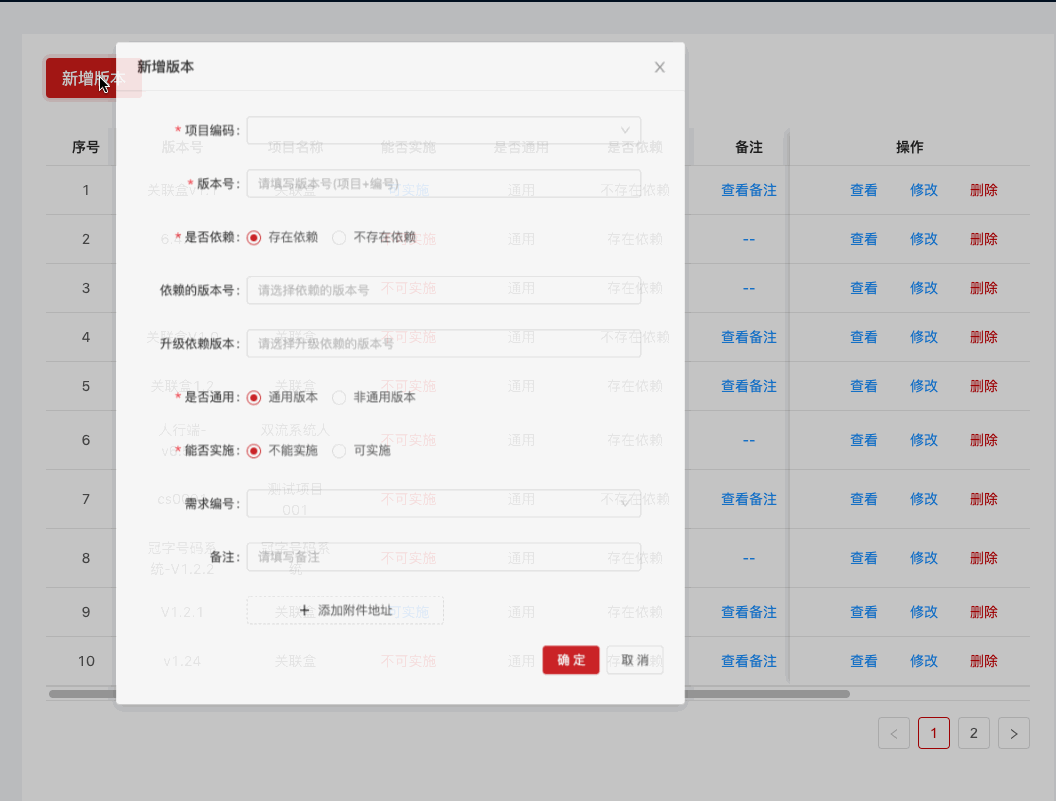
话不多说,上代码
/*
* 创建修改版本
*/
import React from "react";
import { Form, Notification, Button, Input, Row, Col, Select, Radio, Icon } from "antd";
import Request from "@utils/request";
import Storage from "store2";
import "./style.less";
const FormItem = Form.Item;
let id = 0;
class ViewForm extends React.Component {
constructor(props) {
super(props);
this.state = {
versionInfo: {}, // 编辑的初始数据
demandCodeList: [], //需求编号
projectCodeList: [], //项目编号
versionCodeList: [] //依赖的版本编号
};
}
componentDidMount() {
this.getDemandCodeList();
this.getProjectCodeList();
this.getVersionCodeList();
const { taskModalType } = this.props;
if (taskModalType === "edit") {
this.loadData()
}
}
// 获取版本信息
loadData = async () => {
Request.GET(`/api/version/get/${this.props.versionInfo.id}`, {
params: {
loginKey: Storage.get("Authorization"),
}
}).then((res) => {
if (res.success) {
this.setState({
versionInfo: res.data
});
} else {
Notification.warning({
message: res.msg || "获取版本信息失败",
});
}
});
};
// 获取依赖的版本编号列表
getVersionCodeList = () => {
Request.GET("/api/version/list", {
params: {
loginKey: Storage.get("Authorization"),
}
}).then((res) => {
if (res.success) {
this.setState({
versionCodeList: res.data
});
} else {
Notification.warning({
message: res.msg || "获取需求编号列表",
});
}
});
};
// 获取需求编号列表
getDemandCodeList = () => {
Request.GET("/api/demand/list", {
params: {
loginKey: Storage.get("Authorization"),
}
}).then((res) => {
if (res.success) {
this.setState({
demandCodeList: res.data
});
} else {
Notification.warning({
message: res.msg || "获取需求编号列表",
});
}
});
};
// 获取项目列表
getProjectCodeList = () => {
// 获取任务列表
Request.GET("/api/project/list", {
params: {
loginKey: Storage.get("Authorization"),
}
}).then((res) => {
if (res.success) {
this.setState({
projectCodeList: res.data
});
} else {
Notification.warning({
message: res.msg || "获取项目列表失败",
});
}
});
}
handleSubmit = () => {
const { form: { validateFields }, taskModalType } = this.props;
const { versionInfo } = this.state;
validateFields((err, values) => {
if (!err) {
const { names } = values;
console.log("names=",names);
const urlList = names;
if (taskModalType === "edit") {
Request.POST("/api/version/update", {
params: {
loginKey: Storage.get("Authorization"),
},
body: {
"id": versionInfo.id,
"demandCode": values.demandCode,
"dependVersionNumList": values.dependVersionNumList,
"selfDependVersionNumList": values.selfDependVersionNumList,
"projectCode": values.projectCode,
"versionDepend": values.versionDepend,
"versionGeneral": values.versionGeneral,
"versionNum": values.versionNum,
"deployFlag": values.deployFlag,
"note": values.note,
"fileUrlList": urlList
}
}).then((res) => {
if (res.success) {
Notification.success({
message: res.msg || "修改成功",
});
this.props.parentThis.CancelTaskModal();
this.props.parentThis.loadData();
} else {
Notification.warning({
message: res.msg || "修改失败",
});
}
});
} else {
Request.POST("/api/version/add", {
params: {
loginKey: Storage.get("Authorization"),
},
body: {
"demandCode": values.demandCode,
"dependVersionNumList": values.dependVersionNumList,
"selfDependVersionNumList": values.selfDependVersionNumList,
"projectCode": values.projectCode,
"versionDepend": values.versionDepend,
"versionGeneral": values.versionGeneral,
"versionNum": values.versionNum,
"deployFlag": values.deployFlag,
"note": values.note,
"fileUrlList": urlList
}
}).then((res) => {
if (res.success) {
Notification.success({
message: res.msg || "新增版本成功",
});
this.props.parentThis.CancelTaskModal();
this.props.parentThis.loadData();
} else {
Notification.warning({
message: res.msg || "新增版本失败",
});
}
});
}
}
});
};
remove = k => {
const { form } = this.props;
// can use data-binding to get
const keys = form.getFieldValue("keys");
// We need at least one passenger
if (keys.length === 1) {
return;
}
// can use data-binding to set
form.setFieldsValue({
keys: keys.filter(key => key !== k),
});
};
add = () => {
const { form } = this.props;
// can use data-binding to get
const keys = form.getFieldValue("keys");
const nextKeys = keys.concat(id);
id++;
// can use data-binding to set
// important! notify form to detect changes
form.setFieldsValue({
keys: nextKeys,
});
};
render() {
const { getFieldDecorator, getFieldValue } = this.props.form;
const { taskModalType } = this.props;
const { versionInfo, demandCodeList, projectCodeList, versionCodeList } = this.state;
const formItemLayout = {
labelCol: { span: 5 },
wrapperCol: { span: 18 },
};
// 添加附件地址
const formItemLayoutWithOutLabel = {
wrapperCol: {span: 18, offset: 5 },
};
getFieldDecorator("keys", { initialValue: versionInfo.fileUrlList ? versionInfo.fileUrlList : [] });
const keys = getFieldValue("keys") ? getFieldValue("keys") : [];
console.log(versionInfo, keys);
const formItems = keys.map((k, index) => (
<Form.Item
{...(index === 0 ? formItemLayout : formItemLayoutWithOutLabel)}
label={index === 0 ? "附件地址" : ""}
required={false}
key={k}
>
{getFieldDecorator(`names[${index}]`, {
initialValue: Number.isInteger(k) ? "" : k,
validateTrigger: ["onChange", "onBlur"],
rules: [
{
required: true,
whitespace: true,
message: "请输入附件地址",
},
{
pattern: /^(ht|f)tps?:\/\/(([a-zA-Z0-9_-])+(\.)?)*(:\d+)?(\/((\.)?(\?)?=?&?([a-zA-Z0-9_-]|#|%)(\?)?)*)*$/i,
message: "请输入有效的附件地址",
}
],
})(<Input placeholder="请输入有效的附件地址"
style={{ width: "90%", marginRight: 2 }} />)}
{keys.length > 1 ? (
<Icon
className="dynamic-delete-button"
type="minus-circle-o"
onClick={() => this.remove(k)}
/>
) : null}
</Form.Item>
));
return (
<Row>
<Col>
<Form>
<FormItem label="项目编码"
{...formItemLayout}
>
{getFieldDecorator("projectCode", {
initialValue: taskModalType === "edit" ? versionInfo.projectCode : "",
rules: [{ required: true, message: "请选择项目编码!" }],
})(
<Select
showSearch
placeholder="请选择项目编码"
optionFilterProp="children"
filterOption={(input, option) =>
option.props.children.indexOf(input) >= 0
}
>
{
projectCodeList.map((item, index) => {
return <Select.Option
value={item.projectCode}
key={index}
>
{`${item.projectName}-${item.projectCode}`}
</Select.Option>
})
}
</Select>
)}
</FormItem>
<FormItem label="版本号"
{...formItemLayout}
>
{getFieldDecorator("versionNum", {
initialValue: taskModalType === "edit" ? versionInfo.versionNum : "",
rules: [
{ required: true, message: "请填写版本号(项目+编号)!" },
// {
// validator: (rule, value, callback) => {
// if (!/^[\u4e00-\u9fa50-9a-zA-Z.]*$/.test(value)) {
// callback("版本号仅包含汉字数字、英文、和.");
// }
// callback();
// }
// }
],
})(
<Input placeholder="请填写版本号(项目+编号)" />
)}
</FormItem>
<FormItem label="是否依赖"
{...formItemLayout}
>
{getFieldDecorator("versionDepend", {
initialValue: taskModalType === "edit" ? versionInfo.versionDepend : 1,
rules: [{ required: true, message: "请选择是否依赖!" }],
})(
<Radio.Group >
<Radio value={1}>存在依赖</Radio>
<Radio value={2}>不存在依赖</Radio>
</Radio.Group>
)}
</FormItem>
<FormItem label="依赖的版本号"
{...formItemLayout}
>
{getFieldDecorator("dependVersionNumList", {
initialValue: taskModalType === "edit" ? versionInfo.dependVersionNumList : [],
})(
<Select
showSearch
mode="multiple"
placeholder="请选择依赖的版本号"
optionFilterProp="children"
filterOption={(input, option) =>
option.props.children.indexOf(input) >= 0
}
>
{
versionCodeList.map((item, index) => {
return <Select.Option
value={item.versionNum}
key={index}
>
{item.versionNum}
</Select.Option>
})
}
</Select>
)}
</FormItem>
<FormItem label="升级依赖版本"
{...formItemLayout}
>
{getFieldDecorator("selfDependVersionNumList", {
initialValue: taskModalType === "edit" ? versionInfo.selfDependVersionNumList : [],
})(
<Select
showSearch
mode="multiple"
placeholder="请选择升级依赖的版本号"
optionFilterProp="children"
filterOption={(input, option) =>
option.props.children.indexOf(input) >= 0
}
>
{
versionCodeList.map((item, index) => {
return <Select.Option
value={item.versionNum}
key={index}
>
{item.versionNum}
</Select.Option>
})
}
</Select>
)}
</FormItem>
<FormItem label="是否通用"
{...formItemLayout}
>
{getFieldDecorator("versionGeneral", {
initialValue: taskModalType === "edit" ? versionInfo.versionGeneral : 1,
rules: [{ required: true, message: "请选择是否通用!" }],
})(
<Radio.Group >
<Radio value={1}>通用版本</Radio>
<Radio value={2}>非通用版本</Radio>
</Radio.Group>
)}
</FormItem>
<FormItem label="能否实施"
{...formItemLayout}
>
{getFieldDecorator("deployFlag", {
initialValue: taskModalType === "edit" ? versionInfo.deployFlag : 1,
rules: [{ required: true, message: "请选择能否实施!" }],
})(
<Radio.Group >
<Radio value={1}>不能实施</Radio>
<Radio value={2}>可实施</Radio>
</Radio.Group>
)}
</FormItem>
<FormItem label="需求编号"
{...formItemLayout}
>
{getFieldDecorator("demandCode", {
initialValue: taskModalType === "edit" ? versionInfo.demandCode : "",
})(
<Select
showSearch
placeholder="请选择需求编号"
optionFilterProp="children"
filterOption={(input, option) =>
option.props.children.indexOf(input) >= 0
}
>
{
demandCodeList.map((item) => {
return <Select.Option
value={item.demandCode}
key={item.id}
>
{`${item.demandName}-${item.demandCode}`}
</Select.Option>
})
}
</Select>
)}
</FormItem>
<FormItem label="备注"
{...formItemLayout}
>
{getFieldDecorator("note", {
initialValue: taskModalType === "edit" ? versionInfo.note : "",
})(
<Input placeholder="请填写备注" />
)}
</FormItem>
{formItems}
<Form.Item
{...formItemLayoutWithOutLabel}>
<Button type="dashed"
onClick={this.add}
style={{ width: "50%" }}>
<Icon type="plus" /> 添加附件地址
</Button>
</Form.Item>
</Form>
</Col>
<Col style={{ "textAlign": "right" }}>
<Button type="primary"
htmlType="submit"
onClick={() => {
this.handleSubmit()
}}
>确定</Button>
<Button style={{ "marginLeft": 8 }}
onClick={() => {
this.props.parentThis.CancelTaskModal();
}}
>取消</Button>
</Col>
</Row>
);
}
}
const View = Form.create()(ViewForm);
export default View;
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)