- 作者:zzssdd2
- E-mail:zzssdd2@foxmail.com
一、前言
在使用Cortex-M
内核的MCU进行开发时,有时候会因为对内存错误访问等原因造成程序产生异常从而进入HardFaultHandler
错误中断。如果程序结构比较复杂,尤其是运行了RTOS时可能短时间内不易定位异常产生的原因。Segger提供了一种分析CortexM内核芯片HardFault的方法,我在项目中使用后感觉该方法比较实用,本文用来记录该异常分析组件的使用。
二、组件添加
在SEGGER官网的Application Notes页面下提供了该组件的源码和文档
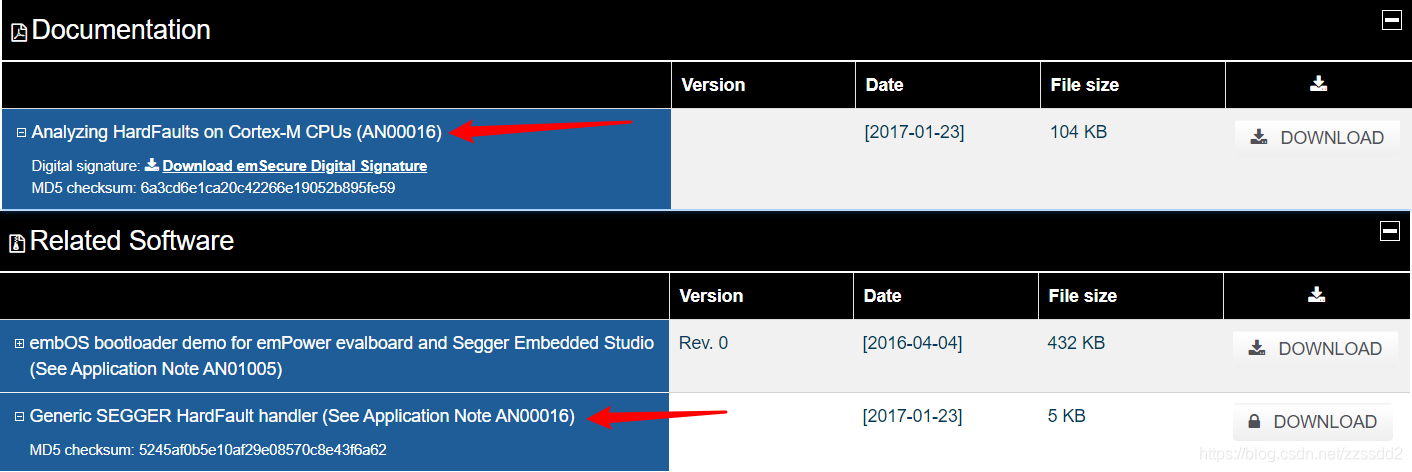
下载下来后将源文件添加到工程中,然后将中断处理文件中的void HardFault_Handler(void)
函数屏蔽掉(和添加的文件中的函数有冲突)就完成添加了。
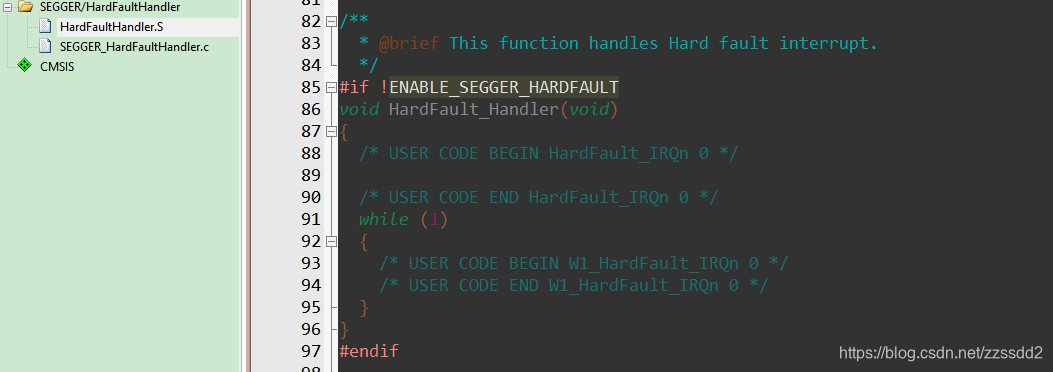
三、组件应用
执行以下代码使程序进入HardFault_Handler
volatile unsigned int* p;
p = (unsigned int*)0x007FFFFF;
*p = 0x123456;
SEGGER_RTT_printf(0, "p = %d\r\n", *p);
在调试模式下运行程序会停在SEGGER_HardFaultHandler.c
文件中的void HardFaultHandler(unsigned int* pStack)
函数中。
void HardFaultHandler(unsigned int* pStack) {
SEGGER_RTT_printf(0, "system enter hard-fault\r\n");
if (NVIC_HFSR & (1u << 31)) {
NVIC_HFSR |= (1u << 31);
*(pStack + 6u) += 2u;
return;
}
#if DEBUG
HardFaultRegs.syshndctrl.byte = SYSHND_CTRL;
HardFaultRegs.mfsr.byte = NVIC_MFSR;
HardFaultRegs.bfsr.byte = NVIC_BFSR;
HardFaultRegs.bfar = NVIC_BFAR;
HardFaultRegs.ufsr.byte = NVIC_UFSR;
HardFaultRegs.hfsr.byte = NVIC_HFSR;
HardFaultRegs.dfsr.byte = NVIC_DFSR;
HardFaultRegs.afsr = NVIC_AFSR;
_Continue = 0u;
while (_Continue == 0u) {
}
HardFaultRegs.SavedRegs.r0 = pStack[0];
HardFaultRegs.SavedRegs.r1 = pStack[1];
HardFaultRegs.SavedRegs.r2 = pStack[2];
HardFaultRegs.SavedRegs.r3 = pStack[3];
HardFaultRegs.SavedRegs.r12 = pStack[4];
HardFaultRegs.SavedRegs.lr = pStack[5];
HardFaultRegs.SavedRegs.pc = pStack[6];
HardFaultRegs.SavedRegs.psr.byte = pStack[7];
_Continue = 0u;
while (_Continue == 0u) {
}
#else
(void)pStack;
do {
} while (1);
#endif
}
在HardFaultRegs
结构体中包含了用来分析异常原因的寄存器
static struct {
struct {
volatile unsigned int r0;
volatile unsigned int r1;
volatile unsigned int r2;
volatile unsigned int r3;
volatile unsigned int r12;
volatile unsigned int lr;
volatile unsigned int pc;
union {
volatile unsigned int byte;
struct {
unsigned int IPSR : 8;
unsigned int EPSR : 19;
unsigned int APSR : 5;
} bits;
} psr;
} SavedRegs;
union {
volatile unsigned int byte;
struct {
unsigned int MEMFAULTACT : 1;
unsigned int BUSFAULTACT : 1;
unsigned int UnusedBits1 : 1;
unsigned int USGFAULTACT : 1;
unsigned int UnusedBits2 : 3;
unsigned int SVCALLACT : 1;
unsigned int MONITORACT : 1;
unsigned int UnusedBits3 : 1;
unsigned int PENDSVACT : 1;
unsigned int SYSTICKACT : 1;
unsigned int USGFAULTPENDED : 1;
unsigned int MEMFAULTPENDED : 1;
unsigned int BUSFAULTPENDED : 1;
unsigned int SVCALLPENDED : 1;
unsigned int MEMFAULTENA : 1;
unsigned int BUSFAULTENA : 1;
unsigned int USGFAULTENA : 1;
} bits;
} syshndctrl;
union {
volatile unsigned char byte;
struct {
unsigned char IACCVIOL : 1;
unsigned char DACCVIOL : 1;
unsigned char UnusedBits : 1;
unsigned char MUNSTKERR : 1;
unsigned char MSTKERR : 1;
unsigned char UnusedBits2 : 2;
unsigned char MMARVALID : 1;
} bits;
} mfsr;
union {
volatile unsigned int byte;
struct {
unsigned int IBUSERR : 1;
unsigned int PRECISERR : 1;
unsigned int IMPREISERR : 1;
unsigned int UNSTKERR : 1;
unsigned int STKERR : 1;
unsigned int UnusedBits : 2;
unsigned int BFARVALID : 1;
} bits;
} bfsr;
volatile unsigned int bfar;
union {
volatile unsigned short byte;
struct {
unsigned short UNDEFINSTR : 1;
unsigned short INVSTATE : 1;
unsigned short INVPC : 1;
unsigned short NOCP : 1;
unsigned short UnusedBits : 4;
unsigned short UNALIGNED : 1;
unsigned short DIVBYZERO : 1;
} bits;
} ufsr;
union {
volatile unsigned int byte;
struct {
unsigned int UnusedBits : 1;
unsigned int VECTBL : 1;
unsigned int UnusedBits2 : 28;
unsigned int FORCED : 1;
unsigned int DEBUGEVT : 1;
} bits;
} hfsr;
union {
volatile unsigned int byte;
struct {
unsigned int HALTED : 1;
unsigned int BKPT : 1;
unsigned int DWTTRAP : 1;
unsigned int VCATCH : 1;
unsigned int EXTERNAL : 1;
} bits;
} dfsr;
volatile unsigned int afsr;
} HardFaultRegs;
将HardFaultRegs
结构体添加到Watch窗口
中,通过一步步向下执行程序可以看到结构体中各参数状态,每个寄存器及寄存器bit位表示什么含义在结构体定义中均有说明。
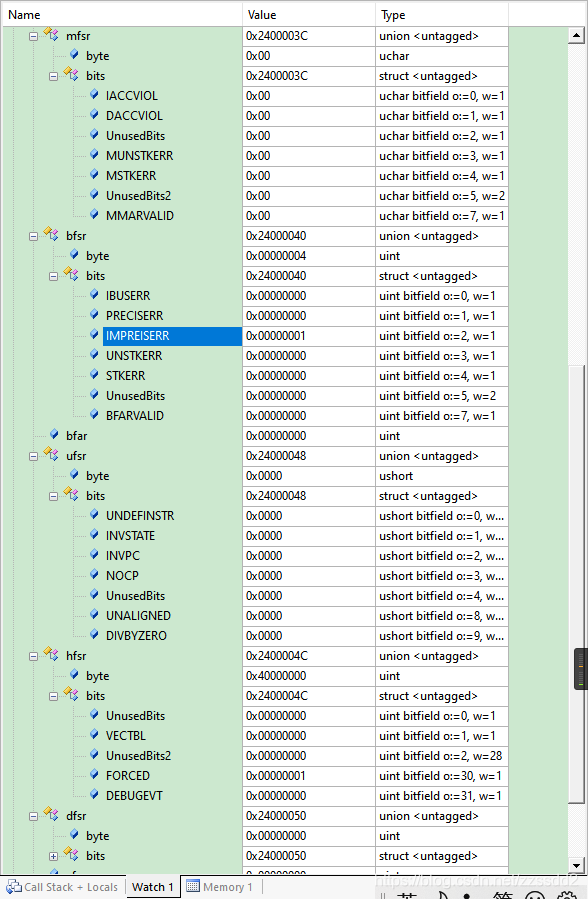
将_Continue
变量也添加到Watch窗口,当执行到以下代码处时在Watch窗口中改变变量值就可以继续向下执行。
_Continue = 0u;
while (_Continue == 0u) {
}

通过对寄存器分析可得出产生异常的原因。
四、扩展
Cortex-M故障异常
Cortex-M processors implement different fault exceptions.
HardFault Exception
The HardFault is the default exception, raised on any error which is not associated with another (enabled) exception.
The HardFault has a fixed priority of -1, i.e. it has a higher priority than all other interrupts and exceptions except for NMI. Therefore a HardFault exception handler can always be entered when an error happens in application code, an interrupt, or another exception. The HardFault is exception number 3 in the vector table with IRQ number -13.
MemManage Exception
The MemManage exception is available with the use of a Memory Protection Unit (MPU) to raise an exception on memory access violations.
The MemManage is exception number 4 in the vector table, IRQ Number -12, and has a configurable priority.
BusFault Exception
The BusFault exception is raised on any memory access error. E.g. by illegal read, write, or vector catch.
The BusFault is exception number 5 in the vector table, IRQ number -11, and has configurable priority. BusFaults can explicitly be enabled in the system control block (SCB). When BusFault is not enabled, a HardFault is raised.
UsageFault Exception
The UsageFault exception is raised on execution errors. Unaligned access on load/store multiple instructions are always caught. Exceptions on other unaligned access, as well as division by zero can be additionally enabled in the SCB.
The UsageFault is exception number 6 in the vector table, IRQ number -10, and has configurable priority. When UsageFault is not enabled, a HardFault is raised instead.
故障状态寄存器
The Cortex-M System Control Block (SCB) contains some registers which enable configuration of exceptions and provide information about faults.
HardFault Status Register (HFSR)
The HFSR is in the SCB at address 0xE000ED2C. It is a 32-bit register.
Bitfields:
[31] DEBUGEVT - Reserved for use by debugger/debug probe. Always write 0.
[30] FORCED - If 1, HardFault has been caused by escalation of another exception, because it is disabled or because of priority.
[1] VECTTBL - If 1, a BusFault occurred by reading the vector table for exception processing.
UsageFault Status Register (UFSR)
The UFSR is a 16-bit pseudo-register, part of the Configurable Fault Status Register (CFSR) at address 0xE000ED28. It can also be directly accessed with halfword access to 0xE000ED2A.
Bitfields:
[9] DIVBYZERO - If 1, SDIV or UDIV instruction executed with divisor 0.
[8] UNALIGNED - If 1, LDM, STM, LDRD, STRD on unaligned address executed, or single load or store executed when enabled to trap.
[3] NOCP - If 1, access to unsupported (e.g. not available or not enabled) coprocessor.
[2] INVPC - If 1, illegal or invalid EXC_RETURN value load to PC.
[1] INVSTATE - If 1, execution in invalid state. E.g. Thumb bit not set in EPSR, or invalid IT state in EPSR.
[0] UNDEFINSTR - If 1, execution of undefined instruction.
BusFault Status Register (BFSR) and BusFault Address Register (BFAR)
The BFSR is a 8-bit pseudo-register in the CFSR. It can be directly accessed with byte access ad 0xE000ED29. The BFAR is a 32-bit register at 0xE000ED38.
Bitfields:
[7] BFARVALID - If 1, the BFAR contains the address which caused the BusFault.
[5] LSPERR - 1f 1, fault during floating-point lazy stack preservation.
[4] STKERR - If 1, fault on stacking for exception entry.
[3] UNSTKERR - If 1, fault on unstacking on exception return.
[2] IMPRECISERR - If 1, return address is not related to fault, e.g. fault caused before.
[1] PRECISERR - If 1, return address instruction caused the fault.
[0] IBUSERR - If 1, fault on instruction fetch.
MemManage Fault Status Register (MMFSR) and MemManage fault Address Register (MMFAR)
The MMFSR is a 8-bit pseudo-register in the CFSR. It can be directly accessed with byte access ad 0xE000ED28. The MMFAR is a 32-bit register at 0xE000ED34.
Bitfields:
[7] MMARVALID - If 1, the MMFAR contains the address which caused the MemManageFault.
[5] MLSPERR - 1f 1, fault during floating-point lazy stack preservation.
[4] MSTKERR - If 1, fault on stacking for exception entry.
[3] MUNSTKERR - If 1, fault on unstacking on exception return.
[1] DACCVIOL - If 1, data access violation.
[0] IACCVIOL - If 1, instruction access violation.
Stack Recovery
On exception entry, the exception handler can check which stack has been used when the fault happened. When bit EXC_RETURN[2] is set, MSP has been used, otherwise PSP has been used.
The stack can be used to recover the CPU register values.
CPU Register Recovery
On exception entry, some CPU registers are stored on the stack and can be read from there for error analysis. the following registers are recoverable:
r0 = pStack[0]; // Register R0
r1 = pStack[1]; // Register R1
r2 = pStack[2]; // Register R2
r3 = pStack[3]; // Register R3
r12 = pStack[4]; // Register R12
lr = pStack[5]; // Link register LR
pc = pStack[6]; // Program counter PC
psr.byte = pStack[7]; // Program status word PSR
故障分析示例
The following examples show how/why some faults can be caused, and how to analyze them. A project to test the faults is available here.
BusFault Examples
Illegal Memory Write
/*********************************************************************
*
* _IllegalWrite()
*
* Function description
* Trigger a BusFault or HardFault by writing to a reserved address.
*
* Additional Information
* BusFault is raised some instructions after the write instruction.
* Related registers on fault:
* HFSR = 0x40000000
* FORCED = 1 - BusFault escalated to HardFault (when BusFault is not activated)
* BFSR = 0x00000004
* IMPREISERR = 1 - Imprecise data access violation. Return address not related to fault
* BFARVALID = 0 - BFAR not valid
*/
static int _IllegalWrite(void) {
int r;
volatile unsigned int* p;
r = 0;
p = (unsigned int*)0x00100000; // 0x00100000-0x07FFFFFF is reserved on STM32F4
// F44F1380 mov.w r3, #0x00100000
*p = 0x00BADA55;
// 4A03 ldr r2, =0x00BADA55
// 601A str r2, [r3] <- Illegal write is done here
return r;
// 9B00 ldr r3, [sp]
// 4618 mov r0, r3
// B002 add sp, sp, #8 <- Fault might be raised here
// 4770 bx lr
}
Illegal Memory Read
/*********************************************************************
*
* _IllegalRead()
*
* Function description
* Trigger a BusFault or HardFault by reading from a reserved address.
*
* Additional Information
* BusFault is immediately triggered on the read instruction.
* Related registers on fault:
* HFSR = 0x40000000
* FORCED = 1 - BusFault escalated to HardFault
* BFSR = 0x00000082
* PRECISERR = 1 - Precise data access violation
* BFARVALID = 1 - BFAR is valid
* BFAR = 0x00100000 - The address read from
*/
static int _IllegalRead(void) {
int r;
volatile unsigned int* p;
p = (unsigned int*)0x00100000; // 0x00100000-0x07FFFFFF is reserved on STM32F4
// F44F1380 mov.w r3, #0x00100000 <- The read address. Will be found in BFAR
r = *p;
// 681B ldr r3, [r3] <- Illegal read happens here and raises BusFault
// 9300 str r3, [sp]
return r;
}
Illegal Function Execution
/*********************************************************************
*
* _IllegalFunc()
*
* Function description
* Trigger a BusFault or HardFault by executing at a reserved address.
*
* Additional Information
* BusFault is triggered on execution at the invalid address.
* Related registers on fault:
* HFSR = 0x40000000
* FORCED = 1 - BusFault escalated to HardFault
* BFSR = 0x00000001
* IBUSERR = 1 - BusFault on instruction prefetch
*/
static int _IllegalFunc(void) {
int r;
int (*pF)(void);
pF = (int(*)(void))0x00100001; // 0x00100000-0x07FFFFFF is reserved on STM32F4
// F44F1380 mov.w r3, #0x00100001
r = pF();
// 4798 blx r3 <- Branch to illegal address, causes fetch from 0x00100000 and fault exception
return r;
}
UsageFault Examples
Undefined Instruction Execution
/*********************************************************************
*
* _UndefInst()
*
* Function description
* Trigger a UsageFault or HardFault by executing an undefined instruction.
*
* Additional Information
* UsageFault is triggered on execution at the invalid address.
* Related registers on hard fault:
* HFSR = 0x40000000
* FORCED = 1 - UsageFault escalated to HardFault
* UFSR = 0x0001
* UNDEFINSTR = 1 - Undefined instruction executed
*/
static int _UndefInst(void) {
static const unsigned short _UDF[4] = {0xDEAD, 0xDEAD, 0xDEAD, 0xDEAD}; // 0xDEAD: UDF #<imm> (permanently undefined)
int r;
int (*pF)(void);
pF = (int(*)(void))(((char*)&_UDF) + 1);
// 4B05 ldr r3, =0x08001C18 <_UDF> <- Load address of "RAM Code" instructions
// 3301 adds r3, #1 <- Make sure Thumb bit is set
r = pF();
// 4798 blx r3 <- Call "RAM Code", will execute UDF instruction and raise exception
// 9000 str r0, [sp]
return r;
}
Illegal State
/*********************************************************************
*
* _NoThumbFunc()
*
* Function description
* Trigger a UsageFault or HardFault by executing an address without thumb bit set.
*
* Additional Information
* UsageFault is triggered on execution at the invalid address.
* Related registers on hard fault:
* HFSR = 0x40000000
* FORCED = 1 - UsageFault escalated to HardFault
* UFSR = 0x0002
* INVSTATE = 1 - Instruction execution with invalid state
*/
static int _NoThumbFunc(void) {
int r;
int (*pF)(void);
pF = (int(*)(void))0x00100000; // 0x00100000-0x07FFFFFF is reserved on STM32F4
// F44F1380 mov.w r3, #0x00100000 <- Note that bit [0] is not set.
r = pF();
// 4798 blx r3 <- Branch exchange with mode change to ARM, but Cortex-M only supports Thumb mode.
return r;
}
Division By Zero
/*********************************************************************
*
* _DivideByZero()
*
* Function description
* Trigger a UsageFault or HardFault by dividing by zero.
*
* Additional Information
* UsageFault is triggered immediately on the divide instruction.
* Related registers on hard fault:
* HFSR = 0x40000000
* FORCED = 1 - UsageFault escalated to HardFault
* UFSR = 0x0200
* DIVBYZERO = 1 - Divide-by-zero fault
*/
static int _DivideByZero(void) {
int r;
volatile unsigned int a;
volatile unsigned int b;
a = 1;
// 2301 movs r3, #1 <- Load dividend
b = 0;
// 2300 movs r3, #0 <- Load divisor
r = a / b;
// FBB2F3F3 udiv r3, r2, r3 <- divide by 0 raises fault exception
return r;
}
Unaligned Access
/*********************************************************************
*
* _UnalignedAccess()
*
* Function description
* Trigger a UsageFault or HardFault by an unaligned word access.
*
* Additional Information
* UsageFault is triggered immediately on the read or write instruction.
* Related registers on fault:
* HFSR = 0x40000000
* FORCED = 1 - UsageFault escalated to HardFault
* UFSR = 0x0100
* UNALIGNED = 1 - Unaligned memory access
*/
static int _UnalignedAccess(void) {
int r;
volatile unsigned int* p;
p = (unsigned int*)0x20000002;
// 4B04 ldr r3, =0x20000002 <- Not word aligned address
r = *p;
// 681B ldr r3, [r3] <- Load word from unaligned address raises exception
// 9300 str r3, [sp]
return r;
}
HardFault Examples
Illegal Vector Table Fetch
/*********************************************************************
*
* _IllegalVector()
*
* Function description
* Trigger a HardFault by interrupt with illegal vector table.
*
* Additional Information
* Related registers on fault:
* HFSR = 0x00000002
* VECTTBL = 1 - Vector table read fault
*/
static int _IllegalVector(void) {
int r;
SCB->VTOR = 0x001000000; // Relocate vector table to illegal address
// 4B09 ldr r3, =0xE000ED00
// F04F7280 mov.w r2, #0x1000000
// 609A str r2, [r3, #8]
SCB->ICSR = SCB_ICSR_PENDSVSET_Msk; // Trigger PendSV exception to read invalid vector
// 4B07 ldr r3, =0xE000ED00
// F04F5280 mov.w r2, #0x10000000
// 605A str r2, [r3, #4]
__ISB();
// F3BF8F6F isb <- PendSV exception is to be executed. PendSV vector is tried to be read from illegal address 0x00100038 causes fault exception
// BF00 nop
__DSB();
// F3BF8F4F dsb sy
// BF00 nop
return r;
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)