文章目录
- Collection接口
- 一、集合框架的概述
- Collection接口继承树
- Collection接口中的方法的使用
- 使用迭代器Iterator遍历Collection集合
- 增强for循环遍历集合
文章 | 链接 |
---|
Java语法 | https://blog.csdn.net/weixin_45606067/article/details/107049186 |
一维数组与二维数组、内存解析 | https://blog.csdn.net/weixin_45606067/article/details/107049178 |
面向对象(1/3)类和对象 | https://blog.csdn.net/weixin_45606067/article/details/108234276 |
面向对象(2/3)封装性、继承性、多态性 | https://blog.csdn.net/weixin_45606067/article/details/108234328 |
面向对象(3/3)抽象类、接口、内部类、代码块 | https://blog.csdn.net/weixin_45606067/article/details/108258152 |
异常处理 | 待更新 |
多线程(1/2) | https://blog.csdn.net/weixin_45606067/article/details/107067785 |
多线程(2/2) | https://blog.csdn.net/weixin_45606067/article/details/107067857 |
常用类 | https://blog.csdn.net/weixin_45606067/article/details/108283203 |
枚举与注解 | 待更新 |
集合(1/5)Collection、Iterator、增强for | https://blog.csdn.net/weixin_45606067/article/details/107046876 |
集合(2/5)List、ArrayList、LinkedList、Vector的底层源码 | https://blog.csdn.net/weixin_45606067/article/details/107069742 |
集合(3/5)set、HashSet、LinkedHashSet、TreeSet的底层源码 | |
集合(4/5)Map、HashMap底层原理分析 | https://blog.csdn.net/weixin_45606067/article/details/107042949 |
集合(5/5)LinkHashMap、TreeMap、Properties、Collections工具类 | https://blog.csdn.net/weixin_45606067/article/details/107069691 |
泛型与File | https://blog.csdn.net/weixin_45606067/article/details/107124099 |
IO流与网络编程 | https://blog.csdn.net/weixin_45606067/article/details/107143670 |
反射机制 | 待更新 |
Java8新特性 | https://blog.csdn.net/weixin_45606067/article/details/107280823 |
Java9/10/11新特性 | 待更新 |
Collection接口
一、集合框架的概述
1.集合、数组都是对多个数据进行存储操作的结构,简称Java容器。
说明:此时的存储,主要指的是内存层面的存储,不涉及持久化存储(.txt,.jpg,.avi,数据库中)
2.数组在存储多个数据方面的特点:
- 一旦初始化以后,其长度就确定了。
- 数组一旦定义好,其元素类型就确定了,我们也就只能操作指定类型的数据。
比如:String[] arr; int[] arr1;Object[] arr2;
数组在存取数据方面的缺点:
- 一旦初始化以后,其长度不可修改。
- 数组中提供的方法非常有限,对于删除、插入数据非常不方便,效率也不高。
- 获取数组中实际元素的个数,数组并没有提供现成的方法。
- 数组存储数据的特点:有序,可重复。
Collection接口继承树
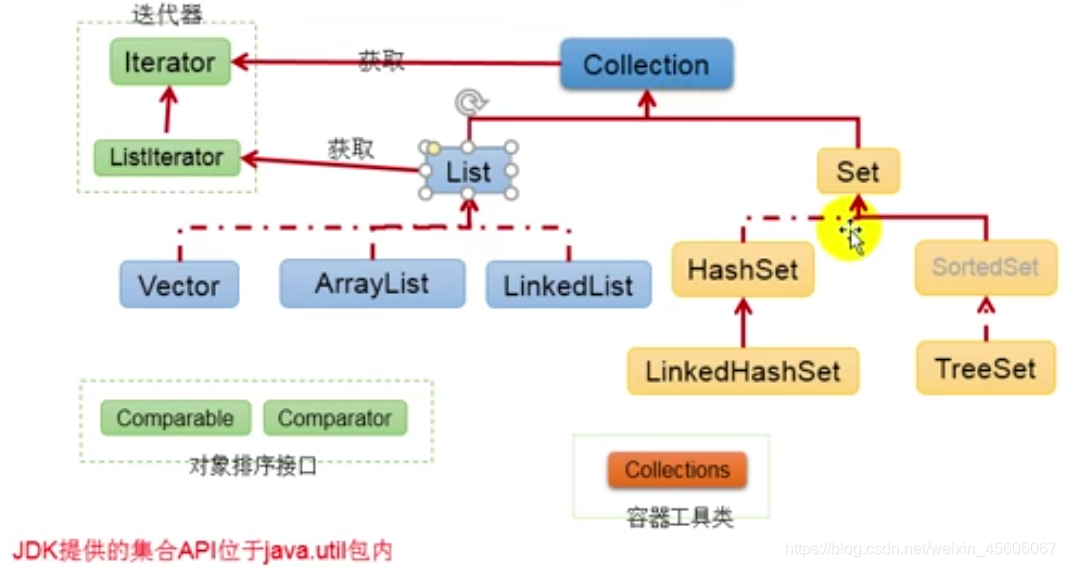
Collection接口中的方法的使用
- add(Object e):将元素e添加到集合coll中
- size():获取添加的元素个数
- addAll(Collection coll1):将coll1集合中的元素添加到当前的集合中
- clear():清空集合元素
- isEmpty():判断当前集合是否为空
代码实现:
@Test
public void test1(){
Collection coll = new ArrayList();
coll.add("AA");
coll.add("BB");
coll.add(123);
coll.add(new Date());
System.out.println(coll.size());
Collection coll1 = new ArrayList();
coll1.add(456);
coll1.add("CC");
coll.addAll(coll1);
System.out.println(coll.size());
System.out.println(coll);
coll.clear();
System.out.println(coll.isEmpty());
}
- contains(Object obj):判断当前集合是否包含obj,在判断时会调用obj对象所在类的equal()。
- containAll(Collection coll1):判断形参coll1中的元素是否都存在于当前集合中。
- remove(Object obj):从当前集合中移除obj元素。返回true/false。
- removeAll(Collection coll2):从当前集合中移除coll2中所有的元素(公共的部分)。
- retainAll(Collection coll3):获取当前集合和coll3集合的交集,并返回给当前集合。
- equals(Object obj):要返回true,需要当前集合与形参集合的元素都相同。
@Test
public void test2(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(456);
coll.add(new String("Tom"));
coll.add(false);
coll.add(new Person("Jerry",20));
boolean contains = coll.contains(123);
System.out.println(contains);
System.out.println(coll.contains(new String("Tom")));
System.out.println(coll.contains(new Person("Jerry",20)));
Collection coll1 = Arrays.asList(123,456);
System.out.println(coll.containsAll(coll1));
coll.remove(123);
Collection coll2 = Arrays.asList(123,4567);
coll.removeAll(coll2);
Collection coll3 = Arrays.asList(123,456,789);
coll.retainAll(coll3);
Collection coll4 = new ArrayList();
coll4.add(123);
coll4.add(456);
coll4.add(new String("Tom"));
coll4.add(false);
coll4.add(new Person("Jerry",20));
coll.equals(coll4);
}
- hashCode():返回当前对象的哈希值
- 集合 —> 数组:toArray()
- 数组 —> 集合:调用Arrays类的静态方法asList()
@Test
public void test3(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(456);
coll.add(new Person("Jerry",20));
coll.add(new String("Tom"));
coll.add(false);
System.out.println(coll.hashCode());
Object[] arr = coll.toArray();
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
List<String> list = Arrays.asList(new String[]{"AA", "BB", "CC"});
System.out.println(list);
List<int[]> list1 = Arrays.asList(new int[]{123, 456});
System.out.println(list1.size());
List<Integer> list2 = Arrays.asList(123, 456);
System.out.println(list2.size());
}
使用迭代器Iterator遍历Collection集合
1.内部的方法:hasNext() 和 next()
2.集合对象每次调用iterator()方法都得到一个全新的迭代器对象,默认游标都在集合的第一个元素之前。
3.内部定义了remove(),可以在遍历的时候,删除集合中的元素。此方法不同于集合直接调用remove()
注意:如果还未调用next()或在上一次调用next方法之后已经调用了remove 方法,再调用remove都会报ILLegalStateException。
代码实现:
public class IteratorTest {
@Test
public void test1(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(456);
coll.add(new Person("Jerry",20));
coll.add(new String("Tom"));
coll.add(false);
Iterator iterator = coll.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
while (iterator.hasNext()){
Object obj = iterator.next();
if ("Tom".equals(obj)){
iterator.remove();
}
}
}
}
迭代器的执行原理
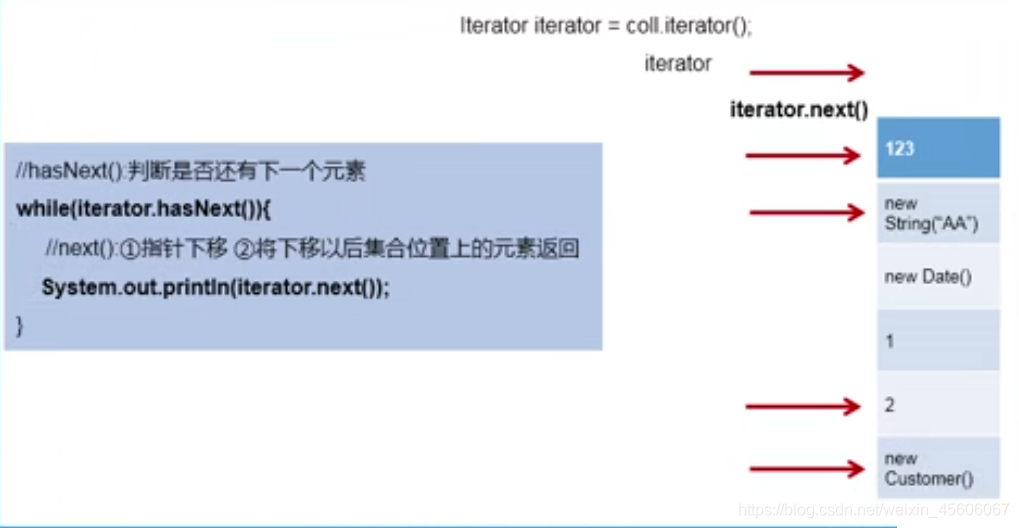
增强for循环遍历集合
JDK5.0 新增了foreach循环,用于遍历集合、数组
定义格式:
for(集合中元素的类型 局部变量 : 集合对象){
sout(局部变量);
}
说明:内部仍然调用了迭代器
把集合中的每个值一次一次赋值给Object类型的变量然后输出
代码实现:
public class forTest {
@Test
public void test1() {
Collection coll = new ArrayList();
coll.add(123);
coll.add(456);
coll.add(new Person("Jerry", 20));
coll.add(new String("Tom"));
coll.add(false);
for (Object o : coll) {
System.out.println(o);
}
}
}
如果有收获!!! 希望老铁们来个三连,点赞、收藏、转发
创作不易,别忘点个赞,可以让更多的人看到这篇文章,顺便鼓励我写出更好的博客
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)