Home location:
http://python.dronekit.io/guide/vehicle_state_and_parameters.html
The Home location isset when a vehicle first gets a good location fix from the GPS. The location isused as the target when the vehicle does a “return to launch”. In Coptermissions (and often Plane) missions, the altitude of waypoints is set relativeto this position.
Vehicle.home_location has the following behavior:
1. In order to get the current value (ina LocationGlobal object) you must first download Vehicle.commands, as shown:
cmds = vehicle.commands
cmds.download()
cmds.wait_ready()
print " Home Location: %s" % vehicle.home_location
The returned value is None before you download the commands or if the home_location has not yet been set by the autopilot. For this reason our example code checks that the value exists (in a loop) before writing it.
# Get Vehicle Home location - will be `None` until first set by autopilot
while not vehicle.home_location:
cmds = vehicle.commands
cmds.download()
cmds.wait_ready()
if not vehicle.home_location:
print " Waiting for home location ..."
# We have a home location.
print "\n Home location: %s" % vehicle.home_location
2. The attribute can be set to a LocationGlobal object (the code fragment below sets it to the current location):
vehicle.home_location=vehicle.location.global_frame
There are some caveats:
- You must be able to read a non-None value before you can write it (the autopilot has to set the value initially before it can be written or read).
- The new location must be within 50 km of the EKF origin or setting the value will silently fail.
- The value is cached in the home_location. If the variable can potentially change on the vehicle you will need to re-download the Vehicle.commands in order to confirm the value.
3. The attribute is not observable.
Note: Vehicle.home_location behaves this way because ArduPilot implements/stores the home location as a waypoint rather than sending them as messages. While DroneKit-Python hides this fact from you when working with commands, to access the value you still need to download the commands.
We hope to improve this attribute in later versions of ArduPilot, where there may be specific commands to get the home locationfrom the vehicle.
Parameters:
1. Getting parameters
# Print the valueof the THR_MIN parameter.
print "Param: %s"% vehicle.parameters['THR_MIN']
2. Setting parameters
# Change theparameter value (Copter, Rover)
vehicle.parameters['THR_MIN']=100
3. Listing all parameters
Vehicle.parameters can be iterated to list all parameters and their values:
print "\nPrintall parameters (iterate `vehicle.parameters`):"
for key, value in vehicle.parameters.iteritems():
print " Key:%s Value:%s" % (key,value)
4. Observing parameter changes
@vehicle.parameters.on_attribute('THR_MIN')
defdecorated_thr_min_callback(self,attr_name, value):
print " PARAMETER CALLBACK: %s changed to: %s" % (attr_name, value)
Taking Off:
Note: Arming turns on the vehicle’s motors inpreparation for flight. The flight controller will not arm until the vehiclehas passed a series of pre-arm checks to ensure that it is safe to fly.
These checks areencapsulated by the Vehicle.is_armable attribute, which is true when the vehicle has booted, EKF is ready, andthe vehicle has GPS lock.
These checks areencapsulated by the Vehicle.is_armable attribute, which is true when the vehicle has booted, EKF is ready, andthe vehicle has GPS lock.
print "Basic pre-arm checks"
# Don't let theuser try to arm until autopilot is ready
whilenot vehicle.is_armable:
print " Waiting for vehicle to initialise..."
time.sleep(1)
If you need more status information you can perform the followingsorts of checks:
if v.mode.name== "INITIALISING":
print "Waiting for vehicle to initialise"
time.sleep(1)
whilevehicle.gps_0.fix_type < 2:
print "Waiting for GPS...:", vehicle.gps_0.fix_type
time.sleep(1)
Velocity control:
The function send_ned_velocity() below generates aSET_POSITION_TARGET_LOCAL_NED MAVLink message which is used to directly specify the speedcomponents of the vehicle in theMAV_FRAME_LOCAL_NED frame (relative to home location). The message is re-sent everysecond for the specified duration.
Note: From Copter 3.3 the vehicle will stop moving if a new messageis not received in approximately 3 seconds. Prior to Copter 3.3 the messageonly needs to be sent once, and the velocity remains active until the nextmovement command is received. The example code works for both cases!
def send_ned_velocity(velocity_x, velocity_y,velocity_z, duration):
"""
Move vehicle in direction based onspecified velocity vectors.
"""
msg = vehicle.message_factory.set_position_target_local_ned_encode(
0, # time_boot_ms(not used)
0, 0, #target system, target component
mavutil.mavlink.MAV_FRAME_LOCAL_NED, # frame
0b0000111111000111, # type_mask (only speeds enabled)
0, 0, 0,# x,y, z positions (not used)
velocity_x, velocity_y,velocity_z, # x, y, z velocity in m/s
0, 0, 0,# x,y, z acceleration (not supported yet, ignored in GCS_Mavlink)
0, 0) #yaw, yaw_rate (not supported yet, ignored in GCS_Mavlink)
# send command to vehicle on 1 Hz cycle
for x in range(0,duration):
vehicle.send_mavlink(msg)
time.sleep(1)
The type_mask parameter is a bitmask that indicates which ofthe other parameters in the message are used/ignored by the vehicle (0 meansthat the dimension is enabled, 1 means ignored). In the example the value0b0000111111000111 is used to enable the velocity components.
In the MAV_FRAME_LOCAL_NED the speed components velocity_x and velocity_y are parallel to the North and East directions (not to the front andside of the vehicle). The velocity_z component isperpendicular to the plane of velocity_x and velocity_y, with apositive value towards the ground, following the right-hand convention. For more information about theMAV_FRAME_LOCAL_NED frame of reference, see this wikipedia article on NED.
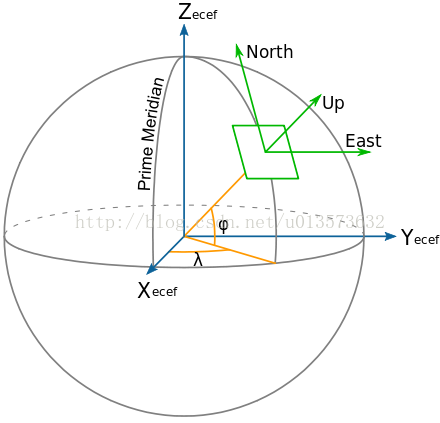
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)