开发的思路
- 布局键盘界面
- 每一个button对应一个槽函数
把输入的字符返回到点击处的文本编辑框
开发过程
首先定义功能button,在头文件中定义
QString getText();
QPushButton *num6Button;
QPushButton *backspaceButton;
QPushButton *num4Button;
QPushButton *okButton;
QPushButton *leftButton;
QPushButton *num1Button;
QPushButton *cancelButton;
QPushButton *rightButton;
QPushButton *num9Button;
QPushButton *num8Button;
QPushButton *num2Button;
QPushButton *num7Button;
QPushButton *dotButton;
QPushButton *num3Button;
QPushButton *num0Button;
QPushButton *num5Button;
QPushButton *signButton;
QLineEdit *lineEdit;
接着定义相关联的槽函数
void on_num1Button_clicked();
void on_num2Button_clicked();
void on_num3Button_clicked();
void on_num4Button_clicked();
void on_num5Button_clicked();
void on_num6Button_clicked();
void on_num7Button_clicked();
void on_num8Button_clicked();
void on_num9Button_clicked();
void on_dotButton_clicked();
void on_num0Button_clicked();
void on_signButton_clicked();
void on_leftButton_clicked();
void on_rightButton_clicked();
void on_backspaceButton_clicked();
void on_cancelButton_clicked();
void on_okButton_clicked();
下面定义界面布局显示的功能模块
okButton = new QPushButton(this);
okButton->setText(" OK");
okButton->setGeometry(QRect(190, 250, 110, 50));
QFont font1;
font1.setPointSize(14);
okButton->setFont(font1);
backspaceButton = new QPushButton(this);
backspaceButton->setText(" backspace");
backspaceButton->setGeometry(QRect(190, 130, 110, 50));
backspaceButton->setFont(font1);
num6Button = new QPushButton(this);
num6Button->setText("6");
num6Button->setGeometry(QRect(130, 130, 50, 50));
QFont font;
font.setPointSize(22);
num6Button->setFont(font);
num4Button = new QPushButton(this);
num4Button->setText(" 4");
num4Button->setGeometry(QRect(10, 130, 50, 50));
num4Button->setFont(font);
leftButton = new QPushButton(this);
leftButton->setText("<-");
leftButton->setGeometry(QRect(190, 190, 50, 50));
leftButton->setFont(font);
num1Button = new QPushButton(this);
num1Button->setText("1");
num1Button->setGeometry(QRect(10, 70, 50, 50));
num1Button->setFont(font);
cancelButton = new QPushButton(this);
cancelButton->setText("Esc");
cancelButton->setGeometry(QRect(190, 70, 110, 50));
cancelButton->setFont(font1);
rightButton = new QPushButton(this);
rightButton->setText("->");
rightButton->setGeometry(QRect(250, 190, 50, 50));
rightButton->setFont(font);
num9Button = new QPushButton(this);
num9Button->setText("9");
num9Button->setGeometry(QRect(130, 190, 50, 50));
num9Button->setFont(font);
num8Button = new QPushButton(this);
num8Button->setText("8");
num8Button->setGeometry(QRect(70, 190, 50, 50));
num8Button->setFont(font);
num2Button = new QPushButton(this);
num2Button->setText("2");
num2Button->setGeometry(QRect(70, 70, 50, 50));
num2Button->setFont(font);
num7Button = new QPushButton(this);
num7Button->setText("7");
num7Button->setGeometry(QRect(10, 190, 50, 50));
num7Button->setFont(font);
dotButton = new QPushButton(this);
dotButton->setText(".");
dotButton->setGeometry(QRect(130, 250, 50, 50));
dotButton->setFont(font);
num3Button = new QPushButton(this);
num3Button->setText("3");
num3Button->setGeometry(QRect(130, 70, 50, 50));
num3Button->setFont(font);
num0Button = new QPushButton(this);
num0Button->setText("0");
num0Button->setGeometry(QRect(10, 250, 50, 50));
num0Button->setFont(font);
num5Button = new QPushButton(this);
num5Button->setText("5");
num5Button->setGeometry(QRect(70, 130, 50, 50));
num5Button->setFont(font);
signButton = new QPushButton(this);
signButton->setText("+/-");
signButton->setGeometry(QRect(70, 250, 50, 50));
QFont font2;
font2.setPointSize(20);
signButton->setFont(font2);
lineEdit = new QLineEdit(this);
lineEdit->setGeometry(QRect(10, 10, 290, 50));
QFont font3;
font3.setPointSize(24);
font3.setWeight(50);
lineEdit->setFont(font3);
lineEdit->setText(QString());
相应的槽函数功能
void NumKeyboard::changeEvent(QEvent *e)
{
QDialog::changeEvent(e);
switch (e->type()) {
case QEvent::LanguageChange:
break;
default:
break;
}
}
bool NumKeyboard::eventFilter(QObject *obj, QEvent *event)
{
if (event->type() == QEvent::KeyPress)
{
QKeyEvent *keyEvent = static_cast<QKeyEvent *>(event);
if (obj == lineEdit)
{
if(keyEvent->key() >= 0x20 && keyEvent->key()<= 0x0ff)
return true;
else
return false;
}
else
{
return false;
}
}
else
{
return QObject::eventFilter(obj, event);
}
}
void NumKeyboard::on_num1Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '1');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
qDebug()<<"strContent"<<strContent;
qDebug()<<"idx"<<idx;
}
void NumKeyboard::on_num2Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '2');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num3Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '3');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num4Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '4');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num5Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '5');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num6Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '6');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num7Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '7');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num8Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '8');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num9Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '9');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_dotButton_clicked()
{
int idx = lineEdit->cursorPosition();
if(idx==0 || strContent.contains('.'))
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '.');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_num0Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0" || (idx==0 &&strContent!=""))
{
lineEdit->setCursorPosition(idx);
qDebug()<<"idx"<<idx;
lineEdit->setFocus();
return;
}
strContent.insert(idx, '0');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_signButton_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(1) == "-")
{
strContent.remove(0, 1);
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx-1);
lineEdit->setFocus();
}
else
{
if(strContent=="0" || strContent=="")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
}
else
{
strContent.insert(0, '-');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
}
}
void NumKeyboard::on_leftButton_clicked()
{
int idx = lineEdit->cursorPosition();
if(idx == 0)
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
lineEdit->setCursorPosition(idx-1);
lineEdit->setFocus();
}
void NumKeyboard::on_rightButton_clicked()
{
int idx = lineEdit->cursorPosition();
if(idx == strContent.length())
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
void NumKeyboard::on_backspaceButton_clicked()
{
int idx = lineEdit->cursorPosition();
if(idx == 0)
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.remove(idx-1,1);
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx-1);
lineEdit->setFocus();
}
void NumKeyboard::on_cancelButton_clicked()
{
this->close();
valid = false;
}
void NumKeyboard::on_okButton_clicked()
{
this->close();
valid = true;
}
void NumKeyboard::setText(QString str)
{
strContent = str;
lineEdit->setText(strContent);
lineEdit->setFocus();
}
QString NumKeyboard::getText()
{
return strContent;
}
上面的槽函数中只对大体的相似,只对不同处做出讲解
以点击“1”为例:
获取在软键盘中的文本框中光标的位置,赋值给idx,索引用
int idx = lineEdit->cursorPosition();
官方的解释如下
QString x = “Pineapple”;
QString y = x.left(4); // y == “Pine”
同理下面的代码含义是:把当前光标位置左侧所有的数,存放在strContent中,
if判断的作用是,当数值开头是0,便不能够输入。一个简单的检查的功能,例如无法输入”01“
insert的官方解释
QString str = “Meal”;
str.insert(1, QString(“ontr”));
// str == “Montreal”
下面语句的作用是在当前的坐标处出插入1;把新的字符串返回给strContent
(初始时idx=0,光标位置是0)
strContent.insert(idx, ‘1’);
随后设置当前的光标的位置+1,
lineEdit->setCursorPosition(idx+1);
显示光标
lineEdit->setFocus();
void NumKeyboard::on_num1Button_clicked()
{
int idx = lineEdit->cursorPosition();
if(strContent.left(idx) == "0")
{
lineEdit->setCursorPosition(idx);
lineEdit->setFocus();
return;
}
strContent.insert(idx, '1');
lineEdit->setText(strContent);
lineEdit->setCursorPosition(idx+1);
lineEdit->setFocus();
}
在设置正负的时候还会用到 strContent.remove(0, 1);
官方的解释是:
QString s = “Montreal”;
s.remove(1, 4);
// s == “Meal”
上面的含义是,在0的位置删除1个字符。就是数字前面的+、-
在设置右移的槽函数中
idx == strContent.length()) //返回此字符串的字符数
最后通过getText() 函数获取文本的内容
QString NumKeyboard::getText() //获取内容
{
return strContent;
}
void SoftKeyLineEdit::mousePressEvent(QMouseEvent *e)
{
if(e->button() == Qt::LeftButton)
{
numkeyboard->setText(this->text());
numkeyboard->exec();
if(numkeyboard->valid)
{
this->setText(numkeyboard->getText());
}
}
}
我们通过鼠标点击的方式来触发软键盘
下面的函数的作用是:把当前的文本框中的内容传递给软件盘中的文本框
numkeyboard->setText(this->text());
下面的exec()与show()显示的方式有所不同。
exec():
显示一个模式对话框,并且锁住程序直到用户关闭该对话框为止。
show():
显示一个非模式对话框。控制权即刻返回给调用函数。
numkeyboard->exec();
如果点击“确认”按键后 valid返回值是true
if(numkeyboard->valid)
随后把返回得到的字符串,传递到点击处的文本框中。
this->setText(numkeyboard->getText());
如何快速一个上面的软键盘到项目中
1.包含上面的cpp和.h文件
2.通过触发的方式触发下面语句
numkeyboard->setText(this->text());
numkeyboard->exec();
if(numkeyboard->valid)
{
this->setText(numkeyboard->getText());
}
3.触发方式可以是信号(例如clicked())
另外看还可以是mousePressEvent(); 它的使用方式要重写
细节(QString 定义的strContent存储有引用计数,指向相同的存储空间,仅仅 是增加一个引用计数)
效果图
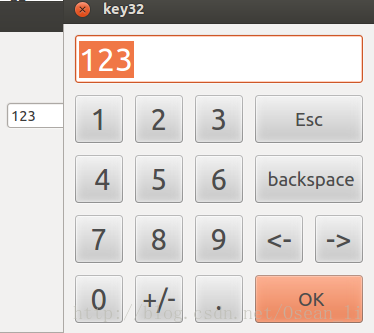
源代码下载
http://download.csdn.net/detail/osean_li/9773460
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)