太久没更新博客了前段时间弄了个计算器
实现了加减乘除复数 带记忆 绘图计算二维图形等功能
先简单讲解下我代码的分块
用图片描述虽然画的丑
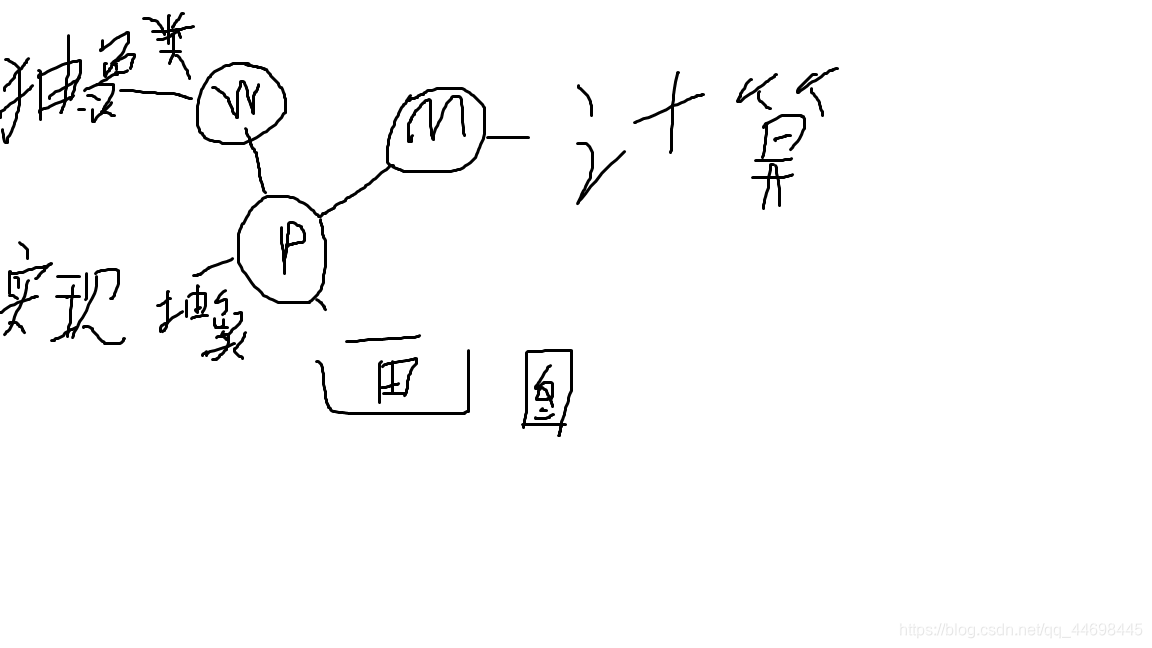
实现效果图片 代码放心使用转载请通知
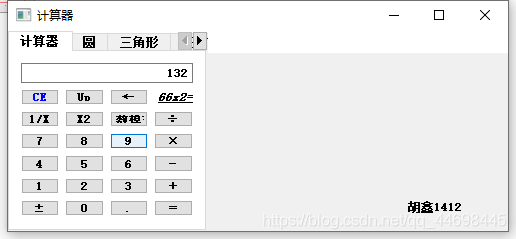
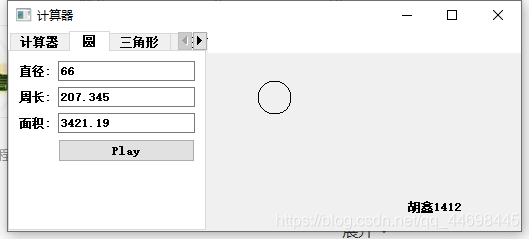
1.Ui
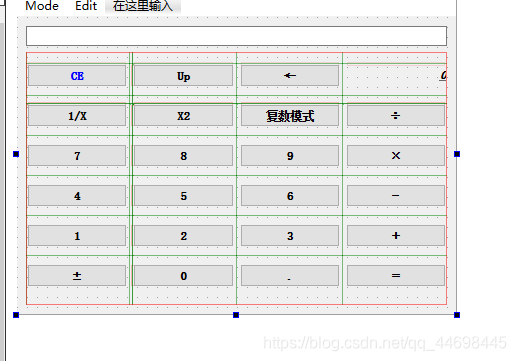
2.在Maindow 类实现计算
maindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include "push.h"
#include <QMainWindow>
#include <QTableWidget>
#include <QLabel>
#include <QRadioButton>
#include <QLineEdit>
#include <QPushButton>
#include <QPainter>
#include <QKeyEvent>
#include <QDebug>
#include <QFormLayout>
#include <QPainterPath>
#include <QtMath>
#include <QStack>
namespace Ui {
class MainWindow;
}
class Comp;
class Push;
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
enum Operator {
NONE,
ADD,
MINUS,
DIV,
MUL
};
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
void pluswait(Operator oper = NONE);
void addwait();
void reducewait();
void ridewait();
void exceptwait();
protected:
void paintEvent(QPaintEvent *event) override;
void keyPressEvent(QKeyEvent *key) override;
private slots:
void on_btn0_clicked();
void on_btn1_clicked();
void on_btn2_clicked();
void on_btn3_clicked();
void on_btn4_clicked();
void on_btn5_clicked();
void on_btn6_clicked();
void on_btn7_clicked();
void on_btn8_clicked();
void on_btn9_clicked();
void on_btnCe_clicked();
void on_btnAdd_clicked();
void on_btnDiv_clicked();
void on_btnDot_clicked();
void on_btnMul_clicked();
void on_btnPop_clicked();
void on_btnCalc_clicked();
void on_btnSign_clicked();
void on_btnClear_clicked();
void on_btnMinus_clicked();
void on_compButton_clicked();
void on_x1Button_clicked();
void on_x2Button_clicked();
void on_leftButton_clicked();
void on_rightButton_clicked();
private:
Ui::MainWindow *ui;
double result;
double mNumber1;
Operator mOperator;
bool mNeedClear;
QString str;
QTabWidget *tab1;
void pushNumber(char n);
void reset();
QString getCurrentNumberStr() const;
int width = 500;
int height = 200;
bool mistype = false;
bool miscomp = false;
Push *push;
Comp *comp1;
Comp *comp2;
QStack<QString>*stack;
QStack<QString>*sta;
};
class Comp{
public:
Comp(double com=0,double con=0):com(com),con(con){}
void setCom(double n) { com = n; }
void setCon(double n) { con = n; }
double getCom() { return com; }
double getCon() { return con; }
Comp *operator+(Comp *comp2){
this->com = this->com+comp2->com;
this->con = this->con+comp2->con;
return this;
}
Comp *operator-(Comp *comp2){
this->com = this->com-comp2->com;
this->con = this->con-comp2->con;
return this;
}
Comp *operator*(Comp *comp2){
this->com = this->com*comp2->com;
this->con = this->con*comp2->con;
return this;
}
Comp *operator/(Comp *comp2){
this->com = this->com/comp2->com;
this->con = this->con/comp2->con;
return this;
}
private:
double com,con;
};
#endif
mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#define PI 3.14159265
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
,push(new Push())
,comp1(new Comp(0,0))
,comp2(new Comp(0,0))
,stack(new QStack<QString>)
,sta(new QStack<QString>)
, mOperator(NONE)
, mNeedClear(false)
{
ui->setupUi(this);
reset();
tab1 = new QTabWidget(this);
tab1->addTab(ui->centralWidget,tr("计算器"));
tab1->addTab(push->getWidget1(),tr("圆"));
tab1->addTab(push->getWidget2(),tr("三角形"));
tab1->addTab(push->getWidget3(),tr("正方形长方形"));
tab1->addTab(push->getWidget4(),tr("梯形"));
tab1->setGeometry(0,0,200,200);
resize(width++,height++);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::pluswait(Operator oper)
{
if(mistype){
double number = ui->edtDisplay->text().toDouble();
mistype = false;
if(number){
switch (mOperator) {
case ADD:
result = mNumber1 + number;
break;
case MINUS:
result = mNumber1 - number;
break;
case MUL:
result = mNumber1 * number;
break;
case DIV:
result = mNumber1 / number;
break;
case NONE:
default:
break;
}
str.append('=');
ui->showlabel->setText(str);
ui->edtDisplay->setText(QString::number(result));
str = str.number(result);
}
}
else {
if(!miscomp && comp1->getCom() && comp1->getCon() && comp2->getCom() && comp2->getCon()){
switch (oper) {
case ADD:comp1->operator+(comp2); break;
case MINUS:comp1->operator-(comp2); break;
case MUL:comp1->operator*(comp2); break;
case DIV:comp1->operator/(comp2); break;
}
ui->edtDisplay->setText(tr("实部:")+QString::number(comp1->getCom())+tr("虚部:")+QString::number(comp1->getCon()));
comp1->setCom(0);
comp1->setCon(0);
comp2->setCom(0);
comp2->setCon(0);
}
}
stack->append(str);
}
void MainWindow::addwait()
{
mOperator = ADD;
mNumber1 = ui->edtDisplay->text().toDouble();
mNeedClear = true;
str.append('+');
ui->edtDisplay->setText(str);
}
void MainWindow::paintEvent(QPaintEvent *event)
{
QPainter paint(this);
QPainterPath path;
if(push->getCurrentPaint() == push->getCurrentRound()){
paint.drawRoundedRect(250,50,push->getmdou()/2,push->getmdou()/2,999,999);
paint.drawText(400,180,tr("胡鑫1412"));
}else if(push->getCurrentPaint() == push->getCurrentTriangle()){
paint.drawLine(250,50,250+push->gettrinw(),50+push->gettrinw());
paint.drawLine(250+push->gettrinw(),50+push->gettrinw(),250-push->gettrinw(),50+push->gettrinw());
paint.drawLine(250-push->gettrinw(),50+push->gettrinw(),250,50);
paint.drawText(400,180,tr("胡鑫1412"));
}else if(push->getCurrentPaint() == push->getCurrentRect()){
paint.drawRect(250,50,push->getrectw(),push->getrectw());
paint.drawText(400,180,tr("胡鑫1412"));
}else if(push->getCurrentPaint() == push->getCurrentMany()){
paint.drawLine(250,50,250+push->getmanyup(),50);
paint.drawLine(250+push->getmanyup(),50,250+push->getmanyh(),50+push->getmanyh());
paint.drawLine(250+push->getmanyh(),50+push->getmanyh(),250-push->getmanydown(),50+push->getmanyh());
paint.drawLine(250-push->getmanydown(),50+push->getmanyh(),250,50);
paint.drawText(400,180,tr("胡鑫1412"));
}
}
void MainWindow::keyPressEvent(QKeyEvent *key)
{
if(key->key() == Qt::Key_Plus && key->modifiers() == Qt::ControlModifier)
addwait();
}
void MainWindow::on_btn0_clicked()
{
pushNumber('0');
}
void MainWindow::on_btn1_clicked()
{
pushNumber('1');
}
void MainWindow::on_btn2_clicked()
{
pushNumber('2');
}
void MainWindow::on_btn3_clicked()
{
pushNumber('3');
}
void MainWindow::on_btn4_clicked()
{
pushNumber('4');
}
void MainWindow::on_btn5_clicked()
{
pushNumber('5');
}
void MainWindow::on_btn6_clicked()
{
pushNumber('6');
}
void MainWindow::on_btn7_clicked()
{
pushNumber('7');
}
void MainWindow::on_btn8_clicked()
{
pushNumber('8');
}
void MainWindow::on_btn9_clicked()
{
pushNumber('9');
}
void MainWindow::on_btnCe_clicked()
{
str = "";
ui->showlabel->setText(str);
reset();
}
void MainWindow::on_btnAdd_clicked()
{
if(!mistype ||!mOperator)
mOperator = ADD;
if(mistype)
pluswait();
else if(!ui->compButton->isChecked() && !mistype){
mNumber1 = ui->edtDisplay->text().toDouble();
mNeedClear = true;
mistype = true;
str.append('+');
}
else {
if(!miscomp && !comp1->getCom()){
comp1->setCom(ui->edtDisplay->text().toDouble());
str.append('+');
miscomp = true;
}
else if(miscomp && !comp1->getCon()){
comp1->setCon(ui->edtDisplay->text().toDouble());
str.append('+');
miscomp = false;
}
else if(!miscomp && !comp2->getCom()){
comp2->setCom(ui->edtDisplay->text().toDouble());
str.append('+');
miscomp = true;
}
else if(miscomp && !comp2->getCon()){
comp2->setCon(ui->edtDisplay->text().toDouble());
miscomp = false;
pluswait(ADD);
}
}
ui->showlabel->setText(str);
}
void MainWindow::on_btnMinus_clicked()
{
if(!mistype ||!mOperator)
mOperator = MINUS;
if(mistype)
pluswait();
else if(!ui->compButton->isChecked() && !mistype){
mNumber1 = ui->edtDisplay->text().toDouble();
mNeedClear = true;
mistype = true;
str.append('-');
}
else {
if(!miscomp && !comp1->getCom()){
comp1->setCom(ui->edtDisplay->text().toDouble());
str.append('-');
miscomp = true;
}
else if(miscomp && !comp1->getCon()){
comp1->setCon(ui->edtDisplay->text().toDouble());
str.append('-');
miscomp = false;
}
else if(!miscomp && !comp2->getCom()){
comp2->setCom(ui->edtDisplay->text().toDouble());
str.append('-');
miscomp = true;
}
else if(miscomp && !comp2->getCon()){
comp2->setCon(ui->edtDisplay->text().toDouble());
miscomp = false;
pluswait(MINUS);
}
}
ui->showlabel->setText(str);
}
void MainWindow::on_btnDiv_clicked()
{
if(!mistype ||!mOperator)
mOperator = DIV;
if(mistype)
pluswait();
else if(!ui->compButton->isChecked() && !mistype){
mNumber1 = ui->edtDisplay->text().toDouble();
mNeedClear = true;
mistype = true;
str.append('/');
}
else {
if(!miscomp && !comp1->getCom()){
comp1->setCom(ui->edtDisplay->text().toDouble());
str.append('/');
miscomp = true;
}
else if(miscomp && !comp1->getCon()){
comp1->setCon(ui->edtDisplay->text().toDouble());
str.append('/');
miscomp = false;
}
else if(!miscomp && !comp2->getCom()){
comp2->setCom(ui->edtDisplay->text().toDouble());
str.append('/');
miscomp = true;
}
else if(miscomp && !comp2->getCon()){
comp2->setCon(ui->edtDisplay->text().toDouble());
miscomp = false;
pluswait(DIV);
}
}
ui->showlabel->setText(str);
}
void MainWindow::on_btnDot_clicked()
{
pushNumber('.');
}
void MainWindow::on_btnMul_clicked()
{
if(!mistype ||!mOperator)
mOperator = MUL;
if(mistype)
pluswait();
else if(!ui->compButton->isChecked() && !mistype){
mNumber1 = ui->edtDisplay->text().toDouble();
mNeedClear = true;
mistype = true;
str.append('x');
}
else {
if(!miscomp && !comp1->getCom()){
comp1->setCom(ui->edtDisplay->text().toDouble());
str.append('x');
miscomp = true;
}
else if(miscomp && !comp1->getCon()){
comp1->setCon(ui->edtDisplay->text().toDouble());
str.append('x');
miscomp = false;
}
else if(!miscomp && !comp2->getCom()){
comp2->setCom(ui->edtDisplay->text().toDouble());
str.append('x');
miscomp = true;
}
else if(miscomp && !comp2->getCon()){
comp2->setCon(ui->edtDisplay->text().toDouble());
miscomp = false;
pluswait(MUL);
}
}
ui->showlabel->setText(str);
}
void MainWindow::on_btnPop_clicked()
{
QString s = ui->edtDisplay->text();
s.remove(s.length()-1,1);
str.remove(s.length(),1);
ui->showlabel->setText(str);
ui->edtDisplay->setText(s);
mNeedClear = false;
}
void MainWindow::on_btnCalc_clicked()
{
if(mOperator == NONE)
return;
qDebug() << str;
if(!ui->compButton->isChecked()){
pluswait();
}
else {
comp2->setCon(ui->edtDisplay->text().toDouble());
miscomp = false;
pluswait(mOperator);
}
mOperator = NONE;
mNeedClear = true;
}
void MainWindow::on_btnSign_clicked()
{
if(mNeedClear) {
reset();
}
str = getCurrentNumberStr();
if(!str.isEmpty() && str.at(0)=='-')
str.remove(0, 1);
else
str.prepend('-');
ui->edtDisplay->setText(str);
}
void MainWindow::on_btnClear_clicked()
{
if(!stack->isEmpty()){
sta->append(stack->top());
str = stack->top();
ui->edtDisplay->setText(stack->top());
ui->showlabel->setText(stack->top());
stack->pop();
}
else{
while (!sta->isEmpty()){
stack->append(sta->top());
sta->pop();
}
}
}
void MainWindow::pushNumber(char n)
{
if(mNeedClear) {
reset();
}
QString numberStr;
if(!ui->compButton->isChecked()){
numberStr = getCurrentNumberStr();
if(n=='0' && numberStr.isEmpty())
return;
}
else {
}
numberStr.append(n);
str += n;
ui->showlabel->setText(str);
ui->edtDisplay->setText(numberStr);
}
void MainWindow::reset()
{
ui->edtDisplay->setText("0");
mNeedClear = false;
}
QString MainWindow::getCurrentNumberStr() const
{
QString numberStr = ui->edtDisplay->text();
if(!numberStr.isEmpty() && numberStr.at(0)=='0')
numberStr.remove(0, 1);
return numberStr;
}
void MainWindow::on_compButton_clicked()
{
miscomp = false;
mistype = false;
}
void MainWindow::on_x1Button_clicked()
{
QString s = ui->edtDisplay->text();
double x1 = s.toDouble();
x1 = 1/x1;
str = str.number(x1);
ui->showlabel->setText(str);
ui->edtDisplay->setText(QString("%1").arg(x1));
}
void MainWindow::on_x2Button_clicked()
{
QString s = ui->edtDisplay->text();
double x2 = s.toDouble();
x2 = x2*x2;
str = str.number(x2);
ui->showlabel->setText(str);
ui->edtDisplay->setText(QString("%1").arg(x2));
}
3.Widget 这个类是继承Widget的
他被我用作一个纯虚类派生给push类实现的
widget.h
#ifndef WIDGET_H
#define WIDGET_H
#include <QLabel>
#include <QRadioButton>
#include <QLineEdit>
#include <QPushButton>
#include <QPainter>
#include <QDebug>
#include <QFormLayout>
#include <QPainterPath>
#include <QtMath>
#include <QWidget>
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
enum Paint{
Round,
Triangle,
Rect,
Many
};
virtual void playRound() = 0;
virtual void playTriangle() = 0;
virtual void playRect() = 0;
virtual void playMany() = 0;
};
#endif
widget.cpp
#include "widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
}
Widget::~Widget()
{
}
5.push类继承widget类 实现里面的虚函数 这里面是绘画类容
push.h
#ifndef PUSH_H
#define PUSH_H
#include "widget.h"
#include <QPaintEvent>
class Push :public Widget
{
Q_OBJECT
public:
Push(QWidget *parent = nullptr);
~Push();
virtual void playRound()override;
virtual void playTriangle()override;
virtual void playRect()override;
virtual void playMany()override;
QWidget *getWidget1(){return widget;}
QWidget *getWidget2(){return widget2;}
QWidget *getWidget3(){return widget3;}
QWidget *getWidget4(){return widget4;}
double getmdou(){ return mdou;};
double gettrinw(){ return trinw;};
double getrectw(){ return rectw;};
double getrecth(){ return recth;};
double getmanyup(){ return manyup;};
double getmanydown(){ return manydown;};
double getmanyh(){ return manyh;};
Paint getCurrentPaint(){ return mpaint;}
Paint getCurrentRound()const{ return Round;}
Paint getCurrentTriangle()const{ return Triangle;}
Paint getCurrentRect()const{ return Rect;}
Paint getCurrentMany()const{ return Many;}
void setCurrentPaint(Paint paint);
protected:
void paintEvent(QPaintEvent *event) override;
private slots:
void slotRound();
void slotTringle();
void slotRect();
void slotMany();
private:
QLabel *roundlabel1;
QLabel *roundlabel2;
QLabel *roundlabel3;
QLineEdit *roundline1;
QLineEdit *roundline2;
QLineEdit *roundline3;
QLineEdit *trinline1;
QLineEdit *trinline2;
QLineEdit *trinline3;
QLineEdit *trinline4;
QLineEdit *rectline1;
QLineEdit *rectline2;
QLineEdit *rectline3;
QLineEdit *rectline4;
QLineEdit *manyline1;
QLineEdit *manyline2;
QLineEdit *manyline3;
QLineEdit *manyline4;
QLineEdit *manyline5;
QPushButton *roundbtn;
QPushButton *trinbtn;
QPushButton *rectbtn;
QPushButton *manybtn;
QWidget *widget;
QWidget *widget2;
QWidget *widget3;
QWidget *widget4;
Paint mpaint;
double mdou;
double trinw;
double rectw;
double recth;
double manyup;
double manydown;
double manyh;
int width = 500;
int height = 200;
};
#endif
push.cpp
#include "push.h"
#define PI 3.14159265
Push::Push(QWidget *parent)
: Widget(parent)
{
widget = new QWidget();
widget2 = new QWidget();
widget3 = new QWidget();
widget4 = new QWidget();
playRound();
playTriangle();
playRect();
playMany();
connect(roundbtn,SIGNAL(clicked()),this,SLOT(slotRound()));
connect(trinbtn,SIGNAL(clicked()),this,SLOT(slotTringle()));
connect(rectbtn,SIGNAL(clicked()),this,SLOT(slotRect()));
connect(manybtn,SIGNAL(clicked()),this,SLOT(slotMany()));
}
Push::~Push()
{
}
void Push::playRound()
{
QFormLayout *form = new QFormLayout(widget);
roundline1 = new QLineEdit(widget);
roundline2 = new QLineEdit(widget);
roundline3 = new QLineEdit(widget);
roundlabel1 = new QLabel(tr("直径:"),widget);
roundlabel2 = new QLabel(tr("周长:"),widget);
roundlabel3 = new QLabel(tr("面积:"),widget);
roundline2->setReadOnly(true);
roundline3->setReadOnly(true);
roundline2->setHidden(true);
roundline3->setHidden(true);
roundlabel2->setHidden(true);
roundlabel3->setHidden(true);
roundbtn = new QPushButton(tr("Play"),widget);
form->addRow(roundlabel1,roundline1);
form->addRow(roundlabel2,roundline2);
form->addRow(roundlabel3,roundline3);
form->addWidget(roundbtn);
}
void Push::playTriangle()
{
QFormLayout *form = new QFormLayout(widget2);
trinline1 = new QLineEdit(widget2);
trinline2 = new QLineEdit(widget2);
trinline3 = new QLineEdit(widget2);
trinline4 = new QLineEdit(widget2);
trinline3->setReadOnly(true);
trinline4->setReadOnly(true);
trinline3->setHidden(true);
trinline4->setHidden(true);
form->addRow(tr("底:"),trinline1);
form->addRow(tr("高:"),trinline2);
form->addRow(tr("周长:"),trinline3);
form->addRow(tr("面积:"),trinline4);
trinbtn = new QPushButton(tr("Play"),widget2);
form->addWidget(trinbtn);
}
void Push::playRect()
{
QFormLayout *form = new QFormLayout(widget3);
rectline1 = new QLineEdit(widget3);
rectline2 = new QLineEdit(widget3);
rectline3 = new QLineEdit(widget3);
rectline4 = new QLineEdit(widget3);
rectline3->setReadOnly(true);
rectline4->setReadOnly(true);
rectline3->setHidden(true);
rectline4->setHidden(true);
form->addRow(tr("长:"),rectline1);
form->addRow(tr("宽:"),rectline2);
form->addRow(tr("周长:"),rectline3);
form->addRow(tr("面积:"),rectline4);
rectbtn = new QPushButton(tr("Play"),widget3);
form->addWidget(rectbtn);
}
void Push::playMany()
{
QFormLayout *form = new QFormLayout(widget4);
manyline1 = new QLineEdit(widget4);
manyline2 = new QLineEdit(widget4);
manyline3 = new QLineEdit(widget4);
manyline4 = new QLineEdit(widget4);
manyline5 = new QLineEdit(widget4);
manyline4->setReadOnly(true);
manyline5->setReadOnly(true);
manyline4->setHidden(true);
manyline5->setHidden(true);
form->addRow(tr("上底"),manyline1);
form->addRow(tr("下底:"),manyline2);
form->addRow(tr("高:"),manyline3);
form->addRow(tr("周长:"),manyline4);
form->addRow(tr("面积:"),manyline5);
manybtn = new QPushButton(tr("Play"),widget4);
form->addWidget(manybtn);
}
void Push::setCurrentPaint(Widget::Paint paint)
{
mpaint = paint;
}
void Push::paintEvent(QPaintEvent *event)
{
QPainter paint(this);
switch (mpaint) {
case Round:
paint.drawRoundedRect(250,50,mdou/2,mdou/2,999,999);
paint.drawText(400,180,tr("胡鑫1412"));
mdou = 0;
break;
case Triangle:
paint.drawLine(250,50,250+trinw,50+trinw);
paint.drawLine(250+trinw,50+trinw,250-trinw,50+trinw);
paint.drawLine(250-trinw,50+trinw,250,50);
paint.drawText(400,180,tr("胡鑫1412"));
trinw = 0;
break;
case Rect:
paint.drawRect(250,50,rectw,recth);
paint.drawText(400,180,tr("胡鑫1412"));
rectw = 0;
recth = 0;
break;
case Many:
paint.drawLine(250,50,250+manyup,50);
paint.drawLine(250+manyup,50,250+manyh,50+manyh);
paint.drawLine(250+manyh,50+manyh,250-manydown,50+manyh);
paint.drawLine(250-manydown,50+manyh,250,50);
paint.drawText(400,180,tr("胡鑫1412"));
manyh = 0;
manyup = 0;
manydown = 0;
break;
default:
break;
}
}
void Push::slotRound()
{
roundline2->setHidden(false);
roundline3->setHidden(false);
roundlabel2->setHidden(false);
roundlabel3->setHidden(false);
QString s = roundline1->text();
mdou = s.toDouble();
double r = mdou/2;
double length = mdou*PI;
double size = PI*(r*r);
roundline2->setText(QString::number(length));
roundline3->setText(QString::number(size));
mpaint = Round;
update();
}
void Push::slotTringle()
{
trinline3->setHidden(false);
trinline4->setHidden(false);
QString s = trinline1->text();
QString s2 = trinline2->text();
trinw = s.toDouble();
double h = s.toDouble();
double length = trinw+trinw+trinw;
double size = (trinw*h)/2;
trinline3->setText(QString::number(length));
trinline4->setText(QString::number(size));
mpaint = Triangle;
update();
}
void Push::slotRect()
{
rectline3->setHidden(false);
rectline4->setHidden(false);
QString s = rectline1->text();
QString s2 = rectline2->text();
rectw = s.toDouble();
recth = s.toDouble();
double length = (rectw+recth)*2;
double size = rectw*recth;
rectline3->setText(QString::number(length));
rectline4->setText(QString::number(size));
mpaint = Rect;
update();
}
void Push::slotMany()
{
manyline4->setHidden(false);
manyline5->setHidden(false);
QString s = manyline1->text();
QString s2 = manyline2->text();
QString s3 = manyline2->text();
manyup = s.toDouble();
manydown = s2.toDouble();
manyh = s3.toDouble();
double length = manyup+manydown+manyh*2;
double size = (manyup+manydown)*manyh/2;
manyline4->setText(QString::number(length));
manyline5->setText(QString::number(size));
mpaint = Many;
update();
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)